Dictionary排序返回只包含键的list
时间: 2024-12-09 22:17:51 浏览: 8
在 C# 中,`Dictionary<TKey, TValue>` 类型并不直接支持按值或键排序,因为它是一个无序的数据结构。但是你可以先将 `Dictionary` 转换为 `KeyValuePair<TKey, TValue>[]` 数组,然后对这个数组进行排序,最后再从排序后的数组中提取键(`TKey`)。
以下是一个示例,展示了如何使用 LINQ 的 `OrderBy` 方法按照键对 `Dictionary` 进行排序,并获取只包含键的列表:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main()
{
// 假设我们有一个 Dictionary 示例
Dictionary<string, int> dict = new Dictionary<string, int>
{
{"Z", 5},
{"A", 1},
{"B", 3}
};
// 将 Dictionary 转换为 KeyValuePair[] 并排序
var sortedItems = dict.OrderBy(pair => pair.Key).ToArray();
// 提取并创建只包含键的 List<TKey>
List<string> keys = sortedItems.Select(item => item.Key).ToList();
Console.WriteLine("Sorted Keys:");
foreach (string key in keys)
{
Console.Write(key + " ");
}
}
}
```
当你运行这段代码时,输出将会是按字母顺序排列的字典键:“A B Z”。
阅读全文
相关推荐
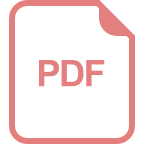
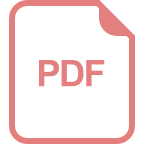
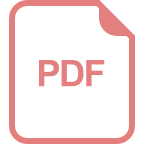
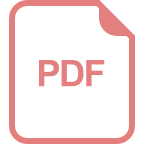
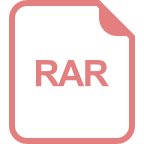
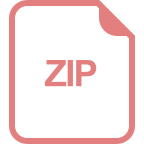
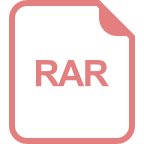
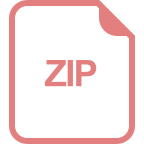
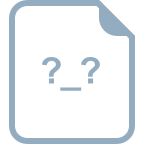
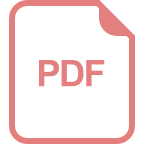
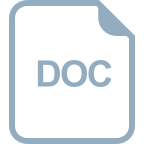
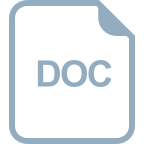
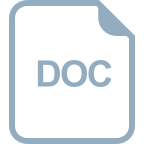
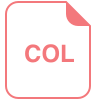
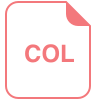
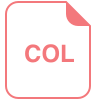
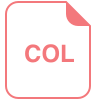

