compute_Wk
时间: 2024-07-06 12:01:14 浏览: 79
`compute_Wk`通常是一个数学或编程中的术语,可能指的是计算某个权重矩阵W在特定时间步k的值。在深度学习或机器学习中,权重矩阵(Weight Matrix)W是神经网络中连接层之间的参数,`compute_Wk`可能是训练过程中更新模型参数的一部分,比如在梯度下降法中计算Wk的新值基于梯度和学习率。
具体来说,这个过程可能涉及到以下几个步骤:
1. 前向传播计算:使用当前的Wk来计算输出,得到预测结果。
2. 计算误差:根据预测结果和真实值之间的差异,计算损失函数。
3. 反向传播:根据损失函数的梯度更新Wk,这可能涉及链式法则和梯度乘以学习率。
4. 更新规则:可能使用随机梯度下降(SGD)、批量梯度下降(BGD)或更复杂的方法(如Adam、RMSprop)来调整Wk的值。
相关问题
compute_loss爆红
根据提供的引用内容,compute_loss爆红可能是由于以下几个原因导致的:
1. compute_loss函数未定义:在代码中使用compute_loss函数,但是没有在代码中定义该函数。这会导致编译器无法找到该函数并报错。
2. compute_loss函数参数错误:如果compute_loss函数的参数与实际调用时的参数不匹配,也会导致编译器报错。请确保函数的参数数量和类型与实际调用时的参数一致。
3. compute_loss函数未导入:如果compute_loss函数所在的模块没有被正确导入,也会导致编译器无法找到该函数并报错。请确保正确导入compute_loss函数所在的模块。
4. compute_loss函数命名冲突:如果compute_loss函数的名称与其他已经定义的函数或变量名称冲突,也会导致编译器报错。请确保compute_loss函数的名称与其他函数或变量名称不冲突。
请检查以上几个原因,找出导致compute_loss爆红的具体原因,并进行相应的修正。
pycharm compute_cost
PyCharm Compute Cost通常是指在PyCharm(Python集成开发环境)中计算某个函数或模型的成本、损失或误差的过程。这在机器学习和深度学习项目中常见,特别是在训练神经网络模型时,`compute_cost`可能是评估模型预测结果与实际标签之间差异的一个函数,比如交叉熵损失(Cross-Entropy Loss)。
在Python中,特别是使用TensorFlow、Keras或PyTorch等库时,可能会有一个自定义的`compute_cost`函数,它接收输入数据、模型预测和真实标签作为参数,然后返回一个衡量模型性能的数值,如均方误差(MSE)、负对数似然(Negative Log Likelihood, NLL)等。
例如,在Keras中,你可能会看到这样的结构:
```python
def compute_cost(y_true, y_pred):
return tf.keras.losses.categorical_crossentropy(y_true, y_pred)
```
如果你正在询问如何在PyCharm中直接使用这个函数,那可能需要设置好项目的路径,导入该函数,并通过运行代码来得到成本值。具体操作取决于你的项目结构和代码布局。
相关推荐
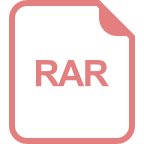
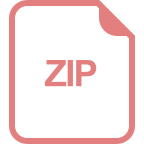
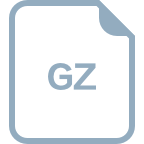












