使用python生成3维空间并打印
时间: 2023-05-12 11:05:47 浏览: 51
可以使用numpy库来生成3维空间,并使用print函数打印出来。以下是示例代码:
```python
import numpy as np
# 生成3维空间
space = np.zeros((3, 3, 3))
# 打印3维空间
print(space)
```
输出结果如下:
```
[[[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]]
[[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]]
[[0. 0. 0.]
[0. 0. 0.]
[0. 0. 0.]]]
```
相关问题
用代码实现随机生成任意三维空间体
生成三维空间体的随机代码取决于您所选择的编程语言,但以下是用 Python 实现的代码示例:
```python
import random
def random_3d_shape():
shape = random.choice(["sphere", "cube", "cylinder", "cone", "pyramid"])
if shape == "sphere":
return "Sphere with radius {} at position ({}, {}, {})".format(
random.uniform(1, 10),
random.uniform(-10, 10),
random.uniform(-10, 10),
random.uniform(-10, 10)
)
elif shape == "cube":
return "Cube with side length {} at position ({}, {}, {})".format(
random.uniform(1, 10),
random.uniform(-10, 10),
random.uniform(-10, 10),
random.uniform(-10, 10)
)
elif shape == "cylinder":
return "Cylinder with radius {} and height {} at position ({}, {}, {})".format(
random.uniform(1, 10),
random.uniform(1, 10),
random.uniform(-10, 10),
random.uniform(-10, 10),
random.uniform(-10, 10)
)
elif shape == "cone":
return "Cone with radius {} and height {} at position ({}, {}, {})".format(
random.uniform(1, 10),
random.uniform(1, 10),
random.uniform(-10, 10),
random.uniform(-10, 10),
random.uniform(-10, 10)
)
elif shape == "pyramid":
return "Pyramid with base length {} and height {} at position ({}, {}, {})".format(
random.uniform(1, 10),
random.uniform(1, 10),
random.uniform(-10, 10),
random.uniform(-10, 10),
random.uniform(-10, 10)
)
print(random_3d_shape())
```
该代码将随机生成一个三维空间体(球体、立方体、圆柱体、圆锥体或金字塔)并打印其位置和大小。
pyomo生成二维变量
要在Pyomo中生成二维变量,你可以使用 `Param` 或 `Var` 组件,并使用两个索引来表示二维空间中的变量。下面是一个示例代码:
```python
from pyomo.environ import *
# 创建一个模型对象
model = ConcreteModel()
# 定义两个索引集合
rows = [1, 2, 3]
cols = [4, 5, 6]
# 定义一个二维参数
model.param = Param(rows, cols, initialize=0)
# 定义一个二维变量
model.var = Var(rows, cols, within=NonNegativeReals)
# 打印参数和变量的值
for i in rows:
for j in cols:
print(f"Parameter ({i}, {j}): {model.param[i, j].value}")
print(f"Variable ({i}, {j}): {model.var[i, j].value}")
```
在这个示例中,我们首先创建了一个模型对象 `model`。然后,定义了两个索引集合 `rows` 和 `cols`,分别表示二维空间中的行和列。
接下来,我们使用 `Param` 组件定义了名为 `param` 的二维参数,使用索引集合 `rows` 和 `cols`,并使用 `initialize` 参数将所有元素初始化为0。
然后,我们使用 `Var` 组件定义了名为 `var` 的二维变量,使用索引集合 `rows` 和 `cols`,并将其定义域设置为非负实数 `NonNegativeReals`。
在打印参数和变量的值时,我们使用 `.value` 属性来获取它们的值。
你可以根据自己的需求修改索引集合、初始化值和定义域。如果需要,还可以使用条件约束等方法对二维变量进行进一步的约束。
希望这个例子能帮助你理解如何生成二维变量!如果还有其他问题,请随时提问。
相关推荐
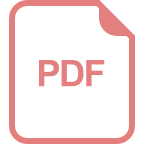












