1. 编程实现:设计一个函数find1(list, x),其中list是列表参数,x是字符串参数。 该函数功能是:在list中查找元素x是否存在,若存在输出'find',若不存在输出'no find'。 测试实例:已知淡水鱼列表['鲤鱼'、'草鱼'、'鲫鱼'、'鳙鱼'、'鲈鱼'],调用函数find()查找'鳊鱼'是否在列表中.
时间: 2023-12-25 13:06:53 浏览: 75
可以使用以下代码实现:
```python
def find1(list, x):
if x in list:
print("find")
else:
print("no find")
# 测试实例
fish_list = ['鲤鱼', '草鱼', '鲫鱼', '鳙鱼', '鲈鱼']
find1(fish_list, '鳊鱼') # 输出:no find
```
运行结果为:no find。因为列表中并没有'鳊鱼'这个元素。
相关问题
、编写程序,要求如下: (1)编写find repeat()函数,该函数从键盘接收一个字符串的输入,然后函数检查该字符串,将其中所有有重复的字符依次打印输出(如有多个,中间以空格隔开),例如:输入“hello world”,则输出“1o”;如果没有重复字符,则输出“无重复字 符”。 (2)编写convert to list()函数,该函数从键盘接收一个字符串的输入,并且将字符串的所有单词拆开成单独的字符串,将所有单词转换为首字母大写的单词后存储到一个列表中,并打印输出该列表。例如:输入为:“Let us go home”,输出为:
对于您的问题,我无法编写和运行代码。但是,我可以给您提供一个参考实现,您可以将其转换为适合您需要的编程语言。
(1)find repeat()函数的参考实现:
```
def find_repeat(s):
counts = {}
repeats = []
for c in s:
counts[c] = counts.get(c, 0) + 1
if counts[c] == 2:
repeats.append(c)
if len(repeats) == 0:
print("无重复字符")
else:
print(" ".join(repeats))
```
(2)convert to list()函数的参考实现:
```
def convert_to_list(s):
words = s.split()
result = []
for word in words:
result.append(word.capitalize())
print(result)
```
您可以在调用这些函数时从键盘获取输入字符串。例如,在Python中,您可以使用以下代码获取输入:
```
s = input("请输入一个字符串:")
find_repeat(s)
convert_to_list(s)
```
3.编写函数listallfiles(path): 形参说明:path是一个文件夹绝对路径, 函数返回值:该文件夹包括子文件夹内所有文件的绝对路径的列表,每个文件的绝对路径是列表的一个元素。 函数功能:谝历指定目录path,将该文件夹包括子文件夹内所有文件的绝对路径写入列表并返回该列表 调用该函数,传递当前文件夹路径做实参,并将调用结果(列表)写入文本文件pathfilespathtxt每个列表元素占一行。 4.编写函数findfiles(path,strkey): 形参说明: path:是一个文件夹绝对路径, strkey:要在文件中查找的字符串。 函数返回值:含有字符串strkey的文本文件列表 函数功能:遍历指定目录绝对路径,将在文件夹包括子文件夹内所有文本文件(txt)中查找包含有字符串 strkey的文件,将含有strkey的文件的绝对路径存入列表并返回该列表。
下面是编写函数listallfiles(path)的代码:
```python
import os
def listallfiles(path):
files = []
for root, dirs, filenames in os.walk(path):
for filename in filenames:
filepath = os.path.join(root, filename)
files.append(filepath)
return files
```
下面是调用该函数并将结果写入文本文件的代码:
```python
path = 'current/directory/path'
files_list = listallfiles(path)
with open('pathfilespathtxt', 'w') as f:
for filepath in files_list:
f.write(filepath + '\n')
```
下面是编写函数findfiles(path, strkey)的代码:
```python
import os
def findfiles(path, strkey):
files = []
for root, dirs, filenames in os.walk(path):
for filename in filenames:
if filename.endswith('.txt'):
filepath = os.path.join(root, filename)
with open(filepath, 'r') as f:
if strkey in f.read():
files.append(filepath)
return files
```
注意,该函数只会查找扩展名为`.txt`的文件。下面是调用该函数的代码:
```python
path = 'current/directory/path'
strkey = 'search_string'
files_list = findfiles(path, strkey)
print(files_list)
```
阅读全文
相关推荐
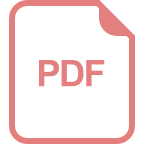
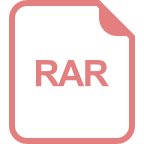
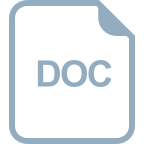
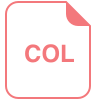
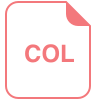










