c# WPF 复选框如何设置在后台类库实时调用checkbox的选中值
时间: 2024-10-24 09:07:58 浏览: 57
在C# WPF中,如果你想在后台类库中实时监听复选框(Checkbox)的选择状态变化,你需要使用`DependencyProperty`以及`INotifyPropertyChanged`接口。以下是步骤:
1. 首先,在你的Checkbox控件上设置一个自定义属性,比如`IsCheckedValue`,作为DependencyProperty:
```csharp
public class MyCheckbox : Control, INotifyPropertyChanged
{
public static readonly DependencyProperty IsCheckedValueProperty =
DependencyProperty.Register("IsCheckedValue", typeof(bool), typeof(MyCheckbox),
new FrameworkPropertyMetadata(false, OnIsCheckedValueChanged));
private bool isChecked;
public bool IsCheckedValue
{
get { return isChecked; }
set
{
if (isChecked != value)
{
isChecked = value;
OnPropertyChanged("IsCheckedValue");
}
}
}
// INotifyPropertyChanged implementation
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
private static void OnIsCheckedValueChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
((MyCheckbox)d).OnPropertyChanged("IsCheckedValue");
}
}
```
2. 在后台类库中订阅这个属性的变化:
```csharp
public class YourBackgroundClass
{
private MyCheckbox checkBox;
public YourBackgroundClass()
{
// 在构造函数中实例化并绑定Checkbox到后台
checkBox = new MyCheckbox();
// 比如你在XAML中这样绑定
// <local:MyCheckbox x:Name="yourCheckbox" IsCheckedValue="{Binding YourCheckboxProperty, Mode=TwoWay}" />
// 当然,这里假设有一个ViewModel类,它有YourCheckboxProperty属性
// DataContext = new ViewModelClass();
checkBox.PropertyChanged += CheckBox_PropertyChanged;
}
private void CheckBox_PropertyChanged(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == ".IsCheckedValue")
{
// 现在你可以访问checkBox.IsCheckedValue获取选中值,并进行相应的处理
bool isChecked = checkBox.IsCheckedValue;
Console.WriteLine($"Checkbox is checked: {isChecked}");
}
}
}
```
每次用户更改了Checkbox的状态,`CheckBox_PropertyChanged`方法就会被调用,你可以在这个方法里获取最新的选中状态。
阅读全文
相关推荐
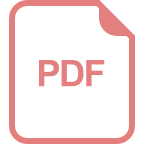
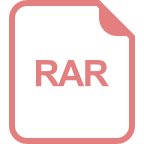
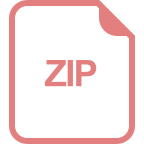







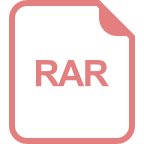
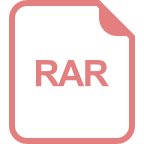
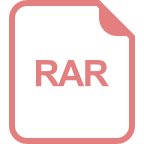
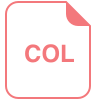
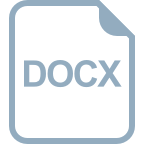