self.files_1 = [] self.files_2 = [] # 获取self.file_path路径下的文件和文件夹 self.items = os.listdir(self.file_path) for item in self.items: # 判断是否为文件 if os.path.isfile(os.path.join(self.file_path, item)): # 判断文件扩展名是否在custom_order列表中 if os.path.splitext(item)[1] in self.custom_order: self.files_1.append(item) # 判断是否为文件夹 elif os.path.isdir(os.path.join(self.file_path, item)): # 打开文件夹并获取其中的文件 folder_path = os.path.join(self.file_path, item) folder_files = [f for f in os.listdir(folder_path) if os.path.isfile(os.path.join(folder_path, f)) and os.path.splitext(f)[1] in self.custom_order] self.files_2.extend(folder_files) # 打印获取到的文件列表 # 对self.files_1按照self.custom_order排序 self.files_1 = sorted(self.files_1, key=lambda x: self.custom_order.index(os.path.splitext(x)[1])) self.files_2 = sorted(self.files_2, key=lambda x: self.custom_order.index(os.path.splitext(x)[1])) print(self.files_1) print(self.files_2)如何获取self.files_2的路径
时间: 2024-04-08 09:30:02 浏览: 14
要获取`self.files_2`中每个文件的完整路径,你可以在添加文件到`self.files_2`列表时保存文件的绝对路径。以下是修改后的代码示例:
```python
self.files_1 = []
self.files_2 = []
self.items = os.listdir(self.file_path)
for item in self.items:
if os.path.isfile(os.path.join(self.file_path, item)):
if os.path.splitext(item)[1] in self.custom_order:
self.files_1.append(item)
elif os.path.isdir(os.path.join(self.file_path, item)):
folder_path = os.path.join(self.file_path, item)
folder_files = [os.path.join(folder_path, f) for f in os.listdir(folder_path) if
os.path.isfile(os.path.join(folder_path, f)) and os.path.splitext(f)[1] in self.custom_order]
self.files_2.extend(folder_files)
self.files_1 = sorted(self.files_1, key=lambda x: self.custom_order.index(os.path.splitext(x)[1]))
self.files_2 = sorted(self.files_2, key=lambda x: self.custom_order.index(os.path.splitext(x)[1]))
print(self.files_1)
print(self.files_2)
```
这样,`self.files_2`列表中的每个元素都是文件的完整路径,包括文件夹路径和文件名。你可以使用`os.path.abspath()`函数获取文件的绝对路径。
相关推荐
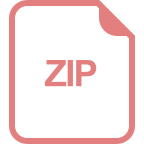
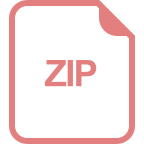
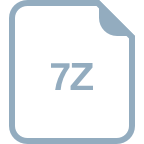














