c++ 连接两个字符串
时间: 2023-12-04 19:05:31 浏览: 31
方法一:使用+号进行字符串拼接
```python
str1 = 'hello'
str2 = 'world'
str3 = str1 + ' ' + str2
print(str3) # 输出:hello world
```
方法二:使用join()方法进行字符串拼接
```python
str1 = 'hello'
str2 = 'world'
str3 = ' '.join([str1, str2])
print(str3) # 输出:hello world
```
方法三:使用f-string进行字符串拼接
```python
str1 = 'hello'
str2 = 'world'
str3 = f'{str1} {str2}'
print(str3) # 输出:hello world
```
相关问题
c++ 将两个字符串连接起来
可以使用字符串拼接符号 "+",例如:
```python
str1 = "Hello"
str2 = "World"
str3 = str1 + str2
print(str3) # 输出 "HelloWorld"
```
也可以使用字符串的 join 方法,例如:
```python
str1 = "Hello"
str2 = "World"
str3 = "".join([str1, str2])
print(str3) # 输出 "HelloWorld"
```
其中,join 方法的参数是一个可迭代对象,例如列表或元组,包含需要连接的字符串。在这个例子中,我们使用了一个空字符串作为连接符,因为我们只需要简单地将两个字符串连接起来。如果需要添加分隔符,则可以在 join 方法中传入相应的字符串,例如:
```python
str1 = "Hello"
str2 = "World"
str3 = "-".join([str1, str2])
print(str3) # 输出 "Hello-World"
```
C++使用指针变量连接两个字符串
下面是一个示例程序,使用指针变量连接两个字符串:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s1[100] = "Hello";
char s2[100] = "World";
char *p = s1 + strlen(s1); // 指向 s1 的末尾
while (*s2) {
*p++ = *s2++; // 将 s2 中的字符拷贝到 s1 的末尾
}
*p = '\0'; // 在连接后的字符串末尾添加空字符
printf("The concatenated string is: %s\n", s1);
return 0;
}
```
运行结果:
```
The concatenated string is: HelloWorld
```
相关推荐
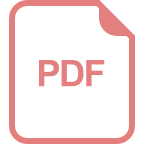












