nestjs socket.io 管理连接、处理事件、广播消息 怎么写
时间: 2024-09-13 19:06:18 浏览: 93
NestJS 是一个用于构建高效、可靠和可扩展的服务器端应用程序的框架。它利用 TypeScript 的强类型特性,采用模块化设计,可以方便地集成不同的后端技术。Socket.IO 是一个用于实时、双向和基于事件的通信的库。它允许服务器端与客户端之间进行实时通信。
在 NestJS 中使用 Socket.IO 管理连接、处理事件和广播消息通常涉及以下步骤:
1. 安装 Socket.IO 相关的库:
首先,你需要安装 `@nestjs/platform-express`(如果你使用的是 Express 作为你的 HTTP 服务器)、`@nestjs/websockets` 和 `socket.io` 相关的包。
```bash
npm install @nestjs/websockets @nestjs/platform-express socket.io
```
2. 创建 WebSocket 模块:
使用 NestJS 的 CLI 或手动创建一个 WebSocket 模块。
```typescript
import { Module } from '@nestjs/common';
import { WebsocketsModule } from '@nestjs/websockets';
@Module({
imports: [WebsocketsModule],
})
export class WebsocketsModule {}
```
3. 创建 Socket 服务:
创建一个服务来处理 Socket 连接、事件监听和广播。
```typescript
import { Injectable } from '@nestjs/common';
import { OnModuleInit, WsResponse, WsResponseFactory } from '@nestjs/websockets';
import { Server } from 'socket.io';
@Injectable()
export class SocketService implements OnModuleInit {
private server: Server;
constructor(private wsResponse: WsResponseFactory) {}
onModuleInit(server: Server) {
this.server = server;
this.server.on('connection', (socket) => {
// 处理新连接
console.log('New client connected:', socket.id);
socket.on('disconnect', () => {
// 处理客户端断开连接
console.log('Client disconnected:', socket.id);
});
});
}
// 自定义事件处理函数
sayHello(client: WsResponse) {
client.emit('hello', 'Hello from server!');
}
// 广播消息给所有客户端
broadcastMessage(message: string) {
this.server.emit('message', message);
}
}
```
4. 配置模块和提供者:
在你的 WebSocket 模块中,配置并提供这个服务。
```typescript
import { Module } from '@nestjs/common';
import { WebsocketsModule } from '@nestjs/websockets';
import { SocketService } from './socket.service';
@Module({
imports: [WebsocketsModule],
providers: [SocketService],
})
export class WebsocketsModule {}
```
5. 在你的主模块中注册 WebSocket 模块:
确保你的 WebSocket 模块在主应用模块中被注册。
```typescript
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
import { WebsocketsModule } from './websockets/websockets.module';
async function bootstrap() {
const app = await NestFactory.create(AppModule);
app.useWebSocketAdapter(new WebsocketsModule().createAdapter(app));
await app.listen(3000);
}
bootstrap();
```
6. 处理事件和广播消息:
在你的服务中定义事件处理函数,然后在客户端监听这些事件。
```typescript
// 客户端(JavaScript)
const socket = io('http://localhost:3000');
socket.on('hello', (message) => {
console.log(message); // 输出: Hello from server!
});
socket.on('message', (message) => {
console.log(message); // 输出: 广播的消息内容
});
// 发送事件给服务器
socket.emit('sayHello', 'Client says hello!');
```
通过以上步骤,你可以在 NestJS 应用中使用 Socket.IO 管理连接、处理事件和广播消息。记得在实际的应用开发中,要遵循安全最佳实践,比如验证客户端消息、限制消息大小等。
阅读全文
相关推荐
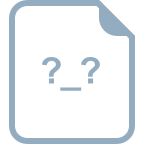
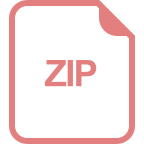
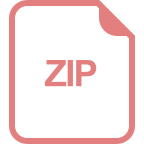
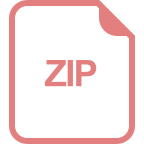
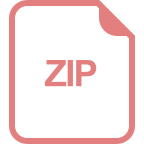
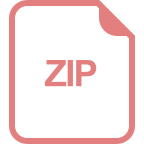
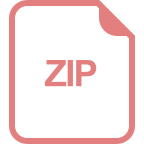
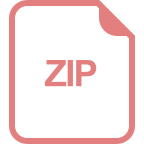
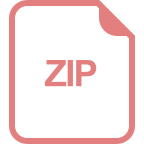
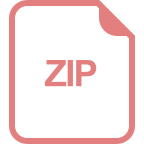
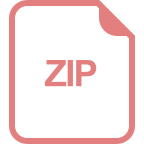
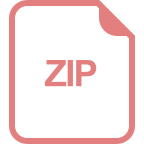
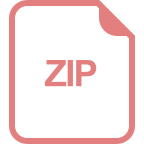
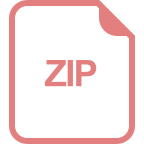
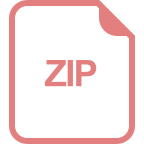
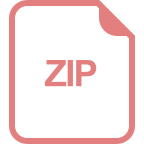
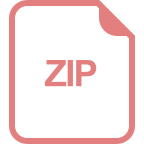
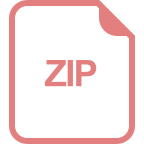
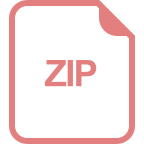