请用C语言编写函数,从文件中读取成绩不及格的学生信息,将结果写入另一个文件。
时间: 2024-05-12 16:18:58 浏览: 65
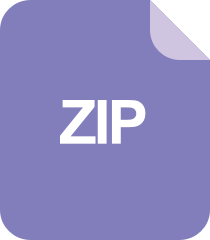
一个用C语言编写的学生信息管理系统,学生数据保存在文本文件中
以下是一个可能的实现:
```c
#include <stdio.h>
typedef struct {
char name[20];
int score;
} Student;
void write_failed_students(const char* input_filename, const char* output_filename) {
FILE* input_file = fopen(input_filename, "r");
if (input_file == NULL) {
printf("Failed to open input file %s\n", input_filename);
return;
}
FILE* output_file = fopen(output_filename, "w");
if (output_file == NULL) {
fclose(input_file);
printf("Failed to open output file %s\n", output_filename);
return;
}
Student student;
while (fscanf(input_file, "%s %d", student.name, &student.score) != EOF) {
if (student.score < 60) {
fprintf(output_file, "%s %d\n", student.name, student.score);
}
}
fclose(input_file);
fclose(output_file);
}
int main() {
write_failed_students("input.txt", "output.txt");
return 0;
}
```
这个程序定义了一个 `Student` 结构体表示学生,包含一个名字和一个分数。`write_failed_students` 函数接受两个文件名参数,打开输入文件并逐行读取学生信息,如果该学生的分数不及格(小于60),则将该学生的信息写入输出文件。最后,两个文件都会被关闭。在 `main` 函数中,我们调用 `write_failed_students` 来执行这个操作。
阅读全文
相关推荐
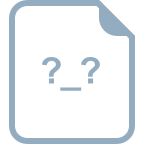




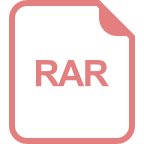
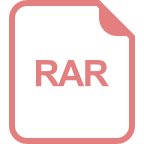
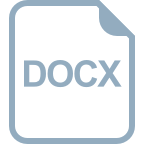
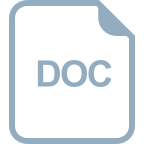
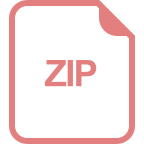
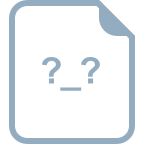
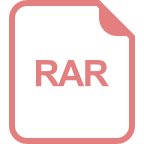
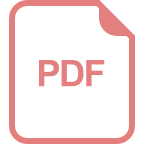
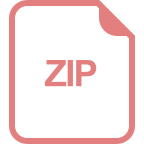
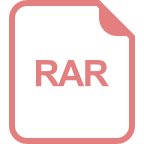
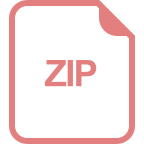
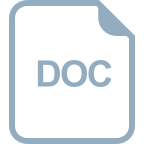