51单片机lcd1602垂直滚动
时间: 2023-07-24 14:03:56 浏览: 38
在51单片机中,LCD1602可以通过编写代码实现垂直滚动。以下是一个简单的示例代码,可以实现LCD1602的垂直滚动:
```
#include<reg51.h>
#include<intrins.h>
#define LCD1602_DB P0
sbit LCD1602_RS = P2^6;
sbit LCD1602_RW = P2^5;
sbit LCD1602_EN = P2^7;
void delay(unsigned int i)
{
while(i--);
}
void write_command(unsigned char command)
{
LCD1602_RS = 0;
LCD1602_RW = 0;
LCD1602_EN = 1;
LCD1602_DB = command;
delay(100);
LCD1602_EN = 0;
}
void write_data(unsigned char data)
{
LCD1602_RS = 1;
LCD1602_RW = 0;
LCD1602_EN = 1;
LCD1602_DB = data;
delay(100);
LCD1602_EN = 0;
}
void init_lcd1602()
{
write_command(0x38);
write_command(0x0c);
write_command(0x06);
write_command(0x01);
}
void write_string(unsigned char x, unsigned char y, unsigned char *string)
{
unsigned char i;
if(x < 16)
{
if(y == 0)
{
write_command(0x80 + x);
}
else if(y == 1)
{
write_command(0x80 + 0x40 + x);
}
for(i = 0; string[i] != '\0'; i++)
{
write_data(string[i]);
}
}
}
void vertical_scroll(unsigned char *string, unsigned char len)
{
unsigned char i, j;
for(i = 0; i < len; i++)
{
for(j = 0; j < 16; j++)
{
write_command(0x80 + j);
write_data(string[i+j]);
}
delay(1000);
}
}
void main()
{
unsigned char string[] = "Hello, World!";
unsigned char len = sizeof(string) - 16;
init_lcd1602();
while(1)
{
vertical_scroll(string, len);
}
}
```
在这个示例中,我们首先定义了一些常量和函数,包括写命令、写数据、初始化LCD1602、写字符串和垂直滚动。然后我们在主函数中初始化LCD1602,并在一个无限循环中调用垂直滚动函数。
在垂直滚动函数中,我们首先遍历字符串中每个字符,并在每次遍历中将字符串中的16个字符写入LCD1602的第一行。然后我们等待一段时间,然后将字符串向左移动一位,继续重复上述操作,直到滚动完整个字符串。
相关推荐
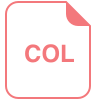
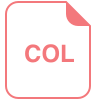











