51单片机LCD1602垂直滚动代码只显示一行
时间: 2023-07-15 16:14:51 浏览: 107
垂直滚动是通过修改LCD DDRAM地址实现的。对于LCD1602,每行显示16个字符,共两行。因此,要实现垂直滚动,需要分别修改第一行和第二行的DDRAM地址指向的位置。
以下是51单片机LCD1602垂直滚动的代码示例:
```
#include <reg52.h>
#include <intrins.h>
#define LCD1602_DB P0
sbit LCD1602_RS = P2^6;
sbit LCD1602_RW = P2^5;
sbit LCD1602_E = P2^7;
void delay(unsigned int i)
{
while(i--);
}
void write_cmd(unsigned char cmd)
{
LCD1602_RS = 0;
LCD1602_RW = 0;
LCD1602_E = 1;
LCD1602_DB = cmd;
_nop_();
_nop_();
_nop_();
LCD1602_E = 0;
}
void write_data(unsigned char dat)
{
LCD1602_RS = 1;
LCD1602_RW = 0;
LCD1602_E = 1;
LCD1602_DB = dat;
_nop_();
_nop_();
_nop_();
LCD1602_E = 0;
}
void init_lcd()
{
write_cmd(0x38); //设置显示模式:16×2显示,5×7点阵字符,8位数据接口
write_cmd(0x0c); //开显示,无光标,不闪烁
write_cmd(0x06); //写入字符后,地址指针自动加1
write_cmd(0x01); //清屏,地址指针指向起始位置
}
void display_string(unsigned char x, unsigned char y, unsigned char *s)
{
unsigned char i;
if(x < 16)
{
if(y == 0) //第一行
{
write_cmd(0x80 + x); //设置DDRAM地址指针到第一行的起始位置+x
}
else if(y == 1) //第二行
{
write_cmd(0xc0 + x); //设置DDRAM地址指针到第二行的起始位置+x
}
for(i = 0; s[i] != '\0'; i++)
{
write_data(s[i]); //逐个写入字符
}
}
}
void scroll_display(unsigned char *s)
{
unsigned char i, j;
while(1)
{
for(i = 0; i < 16; i++) //逐个滚动显示
{
display_string(i, 0, s);
display_string(i, 1, s+16); //第一行和第二行分别显示s和s+16
delay(30000); //滚动速度控制
}
for(j = 0; j < 16; j++) //第一行和第二行分别滚动
{
for(i = 0; i < 15; i++) //先移动15个字符
{
s[i+j] = s[i+j+1]; //字符向前移动一个位置
}
s[i+j] = ' '; //最后一个字符填充空格
display_string(0, 0, s);
display_string(0, 1, s+16); //重新显示
delay(30000); //滚动速度控制
}
}
}
void main()
{
unsigned char str[] = "Hello, world! This is a scrolling message for LCD1602.";
init_lcd();
scroll_display(str);
}
```
注意,此代码中使用了无限循环来实现不断滚动显示。可以根据需要修改滚动次数或添加退出条件。
阅读全文
相关推荐



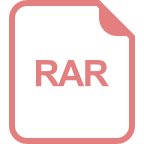
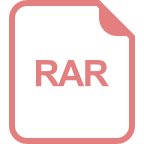
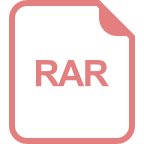
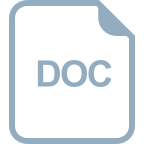
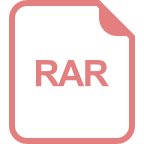
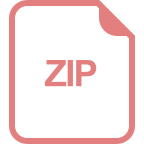
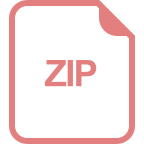
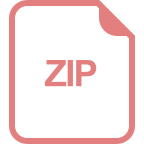