opencv指针式仪表盘读取
时间: 2023-07-18 14:52:15 浏览: 177
读取指针式仪表盘可以通过OpenCV图像处理库来实现。首先,需要通过阈值分割将图像二值化,然后使用霍夫变换检测仪表盘圆形轮廓。接着,可以通过边缘检测和霍夫变换检测仪表盘刻度线的位置,然后计算出指针的位置和角度,最后将指针的角度与仪表盘的刻度对应起来,就可以得到仪表盘的读数。
具体实现可以参考以下步骤:
1. 加载图像并进行灰度化和阈值分割,得到二值化图像。
```python
import cv2
img = cv2.imread('dashboard.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)
```
2. 使用霍夫变换检测仪表盘圆形轮廓。
```python
circles = cv2.HoughCircles(thresh, cv2.HOUGH_GRADIENT, 1, 20, param1=50, param2=30, minRadius=0, maxRadius=0)
```
3. 检测仪表盘刻度线的位置。
```python
edges = cv2.Canny(thresh, 50, 150, apertureSize=3)
lines = cv2.HoughLines(edges, 1, np.pi / 180, 100)
```
4. 计算指针的位置和角度。
```python
for i in circles[0, :]:
center = (i[0], i[1])
radius = i[2]
for line in lines:
rho, theta = line[0]
if abs(theta - np.pi / 2) < np.pi / 6:
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
if x1 != x2:
k = (y2 - y1) / (x2 - x1)
b = y1 - k * x1
x = int((center[1] - b) / k)
y = center[1]
else:
x = x1
y = int(center[1] - (x1 - center[0]) / np.tan(theta))
cv2.line(img, (center[0], center[1]), (x, y), (0, 0, 255), 2)
angle = np.arctan2(y - center[1], x - center[0]) * 180 / np.pi
if angle < 0:
angle += 360
print('Pointer angle:', angle)
```
5. 将指针的角度与仪表盘的刻度对应起来,得到读数。
具体的刻度转换方法需要根据具体的仪表盘设计来确定,可以根据实际情况进行调整。
阅读全文
相关推荐
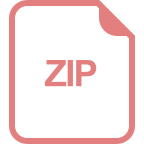

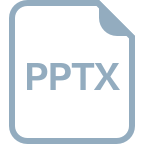
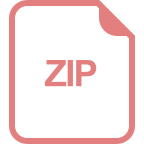
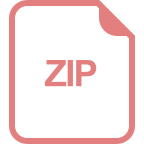
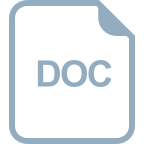

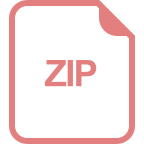
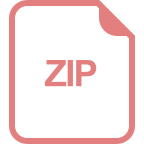
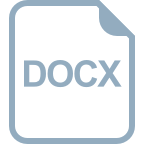
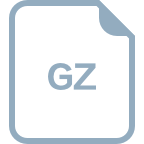
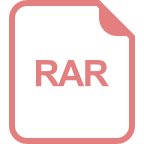
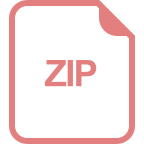
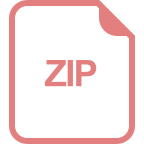
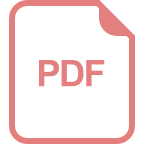
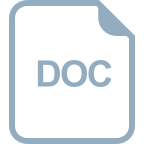