linux环境c语言编程使用匿名管道从父进程向子进程发送你自己的学号,子进程收到后用另一个管道向父进程发回你自己的姓名;对收到的数据要显示到屏幕
时间: 2023-05-28 15:04:35 浏览: 102
以下是一个实现该功能的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define MAX_BUF_SIZE 256
int main(void) {
int fd1[2], fd2[2];
pid_t pid;
char buf[MAX_BUF_SIZE];
if (pipe(fd1) < 0 || pipe(fd2) < 0) {
perror("pipe");
exit(EXIT_FAILURE);
}
if ((pid = fork()) < 0) {
perror("fork");
exit(EXIT_FAILURE);
} else if (pid > 0) { // 父进程
close(fd1[0]); // 关闭读端
close(fd2[1]); // 关闭写端
char student_id[] = "2021000000";
printf("[Parent] My student ID is: %s\n", student_id);
if (write(fd1[1], student_id, strlen(student_id)) < 0) {
perror("write");
exit(EXIT_FAILURE);
}
if (read(fd2[0], buf, MAX_BUF_SIZE) < 0) {
perror("read");
exit(EXIT_FAILURE);
}
printf("[Parent] Received name from child: %s\n", buf);
close(fd1[1]); // 关闭写端
close(fd2[0]); // 关闭读端
} else { // 子进程
close(fd1[1]); // 关闭写端
close(fd2[0]); // 关闭读端
if (read(fd1[0], buf, MAX_BUF_SIZE) < 0) {
perror("read");
exit(EXIT_FAILURE);
}
printf("[Child] Received student ID from parent: %s\n", buf);
char name[] = "Alice";
printf("[Child] My name is: %s\n", name);
if (write(fd2[1], name, strlen(name)) < 0) {
perror("write");
exit(EXIT_FAILURE);
}
close(fd1[0]); // 关闭读端
close(fd2[1]); // 关闭写端
}
return 0;
}
```
该程序首先创建两个管道,分别用于父进程向子进程发送学号和子进程向父进程发送姓名。然后通过 `fork` 创建子进程。
在父进程中,先将自己的学号打印到屏幕上,然后将学号写入管道 `fd1` 的写端。接着从管道 `fd2` 的读端读取子进程发送的姓名,并打印到屏幕上。
在子进程中,先从管道 `fd1` 的读端读取父进程发送的学号,并打印到屏幕上。然后将自己的姓名打印到屏幕上,并将姓名写入管道 `fd2` 的写端。
最后,父进程和子进程分别关闭不需要的管道端,程序结束。
阅读全文
相关推荐
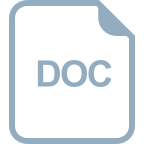
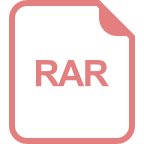
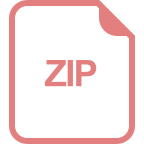
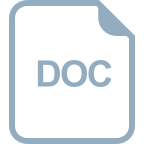
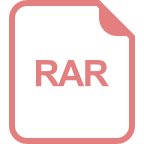
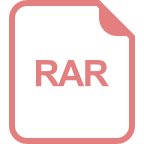
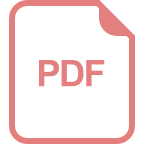
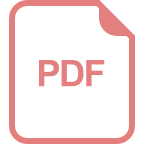
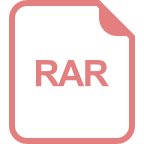
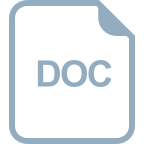
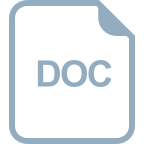