请问 QT 如何读取一个json格式数据,并通过TCP协议发送
时间: 2023-07-19 22:19:49 浏览: 266
在Qt中,你可以使用QJsonDocument类来读取JSON格式的数据。下面是一个简单的例子,用于读取JSON格式的数据并通过TCP协议发送:
```cpp
#include <QJsonDocument>
#include <QJsonObject>
#include <QTcpSocket>
void sendJsonData()
{
// 读取 JSON 数据
QFile file("data.json");
file.open(QIODevice::ReadOnly | QIODevice::Text);
QByteArray jsonData = file.readAll();
QJsonDocument jsonDoc = QJsonDocument::fromJson(jsonData);
QJsonObject jsonObject = jsonDoc.object();
// 创建 TCP 连接
QTcpSocket socket;
socket.connectToHost("example.com", 1234);
if (socket.waitForConnected()) {
// 将 JSON 数据转换为字节数组
QByteArray jsonBytes = jsonDoc.toJson();
// 发送 JSON 数据
qint64 bytesSent = socket.write(jsonBytes);
if (bytesSent == -1) {
// 处理错误
}
// 等待接收响应
if (socket.waitForReadyRead()) {
QByteArray responseData = socket.readAll();
// 处理响应数据
} else {
// 处理错误
}
} else {
// 处理连接错误
}
socket.close();
}
```
在上面的代码中,我们首先使用`QFile`类读取了一个JSON文件,并使用`QJsonDocument`类将其解析为`QJsonObject`对象。接下来,我们创建了一个`QTcpSocket`对象,并使用`connectToHost`方法连接到了远程主机。一旦连接建立成功,我们将JSON对象转换为字节数组,并使用`write`方法将其发送出去。在发送完成后,我们调用`waitForReadyRead`方法等待接收响应数据,并使用`readAll`方法读取响应数据。最后,我们关闭了TCP连接。
阅读全文
相关推荐
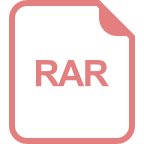
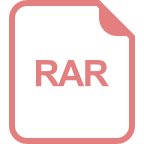
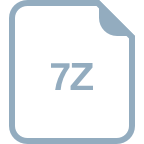
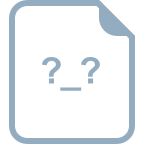
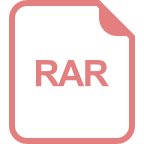
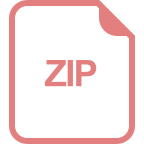
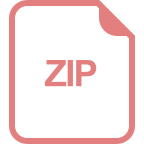
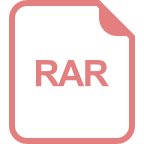
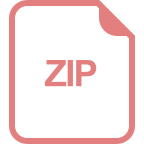
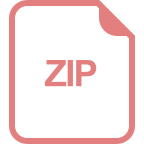
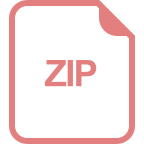
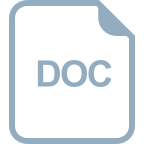
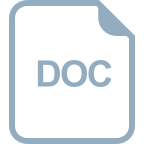


