使用c++解压gzip字节字符串
时间: 2023-08-20 12:13:20 浏览: 134
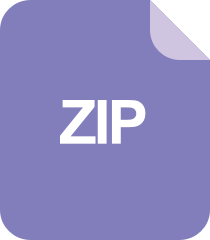
C++解压zip
使用C++解压Gzip字节字符串可以使用zlib库。以下是一个简单的示例代码:
```c++
#include <iostream>
#include <string>
#include <cstring>
#include <zlib.h>
std::string decompressGzip(const char* compressedData, int compressedSize)
{
const int bufferSize = 1024;
char buffer[bufferSize];
z_stream zs;
memset(&zs, 0, sizeof(zs));
if (inflateInit2(&zs, 16 + MAX_WBITS) != Z_OK) {
return "";
}
zs.avail_in = compressedSize;
zs.next_in = (Bytef*)compressedData;
zs.avail_out = bufferSize;
zs.next_out = (Bytef*)buffer;
std::string output;
int status;
do {
status = inflate(&zs, Z_FINISH);
if (output.size() < zs.total_out) {
output.append(buffer, zs.total_out - output.size());
}
zs.avail_out = bufferSize;
zs.next_out = (Bytef*)buffer;
} while (status == Z_OK);
inflateEnd(&zs);
if (status != Z_STREAM_END) {
return "";
}
return output;
}
int main()
{
const char* compressedData = "\x1f\x8b\x08\x00\x00\x00\x00\x00\x02\xff\xcb\x48\xcd\xc9\xc9\x07\x00\x86\x84\x02\x00\x00\x00";
int compressedSize = 23;
std::string uncompressedData = decompressGzip(compressedData, compressedSize);
std::cout << uncompressedData << std::endl;
return 0;
}
```
这个示例代码使用zlib库中的inflate函数对Gzip字节字符串进行解压缩,并返回解压缩后的字符串。在解压缩之前需要调用inflateInit2函数进行初始化,并在解压缩完成后调用inflateEnd函数进行清理。
阅读全文
相关推荐



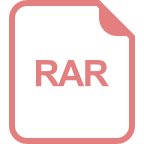
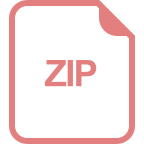
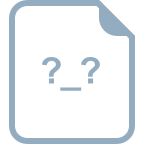
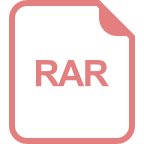
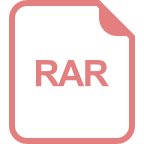
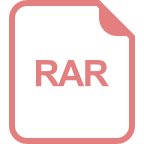
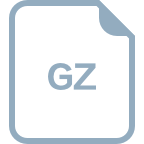
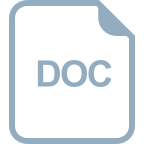
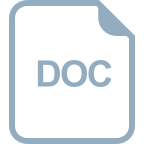

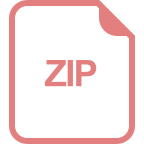