如何解决这个错误if href.endswith('.pdf'): AttributeError: 'NoneType' object has no attribute 'endswith'
时间: 2023-06-13 20:05:45 浏览: 239
这个错误是因为你在一个空的变量(NoneType)上调用了一个方法(endswith)。通常情况下,这是因为你没有检查一个变量是否为空,就尝试对它进行操作。
要解决这个错误,你需要先检查变量是否为空,然后再尝试调用方法。你可以使用以下代码来解决这个问题:
```python
if href is not None and href.endswith('.pdf'):
# do something
```
这里,我们首先检查 href 是否为 None,如果不是,才尝试调用 endswith 方法。这样就可以避免出现以上错误。
相关问题
AttributeError: 'NoneType' object has no attribute 'endswith'
该错误是由于尝试在一个值为`None`的变量上调用`endswith`方法引起的。`NoneType`是Python中表示空值的类型。`endswith`是一个字符串方法,用于检查字符串是否以指定的后缀结尾。但是如果你尝试在一个空值上调用这个方法,就会出现`AttributeError`。
为了解决这个问题,你需要确保变量不是`None`,并且是一个字符串类型才能调用`endswith`方法。你可以使用条件语句来检查变量是否为`None`,并且在调用方法之前进行检查。
例如:
```python
text = None # 假设这是一个空值
if text is not None and isinstance(text, str):
if text.endswith('.txt'):
print('文件名以 .txt 结尾')
else:
print('文件名不以 .txt 结尾')
else:
print('变量为空或不是字符串类型')
```
在上面的示例中,我们首先检查`text`是否为`None`并且是字符串类型。然后在调用`endswith`方法之前,我们再次检查文件名是否以`.txt`结尾。如果变量为空或者不是字符串类型,则会输出相应的错误信息。
attributeerror: 'nonetype' object has no attribute 'endswith'
### 回答1:
这是一个错误提示,意思是“'NoneType'对象没有'endswith'属性”。这通常是因为你在尝试使用一个空对象或None对象的属性或方法,而这个属性或方法不存在。你需要检查你的代码,找出哪个对象是None,然后确保它不是None或者在使用它之前进行判断。
### 回答2:
AttributeError是Python中常见的错误类型之一,表示对象没有指定的属性或方法。'NoneType' object has no attribute 'endswith'的错误通常发生在尝试在None对象上调用endswith()方法时。
在Python中,None是表示空或不存在对象的特殊值。如果尝试在None对象上调用以字符串结尾的endswith()方法,Python会抛出AttributeError异常,提示此对象没有该属性。
为了解决该问题,我们需要确保调用对象不是None。这可以通过添加if语句或使用try-except块来实现。以下是一些解决方法:
方法一:使用if语句
```
string = None
if string:
if string.endswith('word'):
print('string ends with word')
else:
print('string does not end with word')
else:
print('string is None')
```
在上面的代码中,我们首先检查string是否为None,如果不是None,我们再检查是否以指定字符串结尾。如果是,我们输出“string ends with word”,否则,输出“string does not end with word”。如果string是None,输出“string is None”。
方法二:使用try-except块
```
string = None
try:
if string.endswith('word'):
print('string ends with word')
else:
print('string does not end with word')
except AttributeError:
print('string is None')
```
在上面的代码中,我们试图在string上调用endswith()方法,如果该方法不存在,则会引发AttributeError异常。我们使用try-except块来捕获此异常并输出“string is None”。
总结:
'NoneType' object has no attribute 'endswith'的错误通常发生在尝试在None对象上调用endswith()方法时。在编写代码时,应该始终检查变量是否为None,避免出现此类错误。如果无法避免,我们可以使用if语句或try-except块来处理异常。
### 回答3:
这个错误信息通常表示在程序中出现了一个 NoneType 类型对象,但是对该对象执行了一个字符串操作中的 endswith() 方法,而 NoneType 类型对象并没有该方法,所以会抛出 AttributeError 异常。
通常出现这个错误的原因可能是变量为空或者数据类型不一致。在Python中,如果变量没有被赋值或者被赋值为 None,那么该变量的类型就是 NoneType。例如,如果我们使用一个未定义的变量,或者在函数中没有明确返回任何值,那么就会得到一个 NoneType 类型的对象。
为了避免这个错误的出现,我们可以在代码中使用一些条件判断语句,以避免对无效的对象执行操作。例如,我们可以在使用 endswith() 方法之前先判断该对象是否为空或者该对象是否是字符串类型。
另外,如果我们确定变量的值必定不为空,并且需要对其进行字符串操作,那么我们可以使用 Python 的 assert 语句来确认该变量不为空。例如:
``` python
my_str = get_a_string()
assert my_str is not None, "my_str should not be None"
if my_str.endswith("abc"):
print("my_str ends with abc")
```
在这个例子中,如果 my_str 的值为 None,那么就会抛出 AssertionError 异常,并且触发 assert 语句后面的错误信息。但是,如果 my_str 的值不为空,那么程序会正常执行,并且判断该字符串是否以 "abc" 结尾。
阅读全文
相关推荐
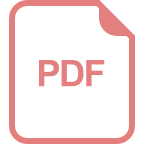
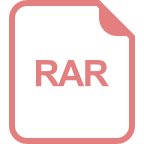
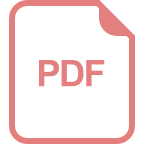



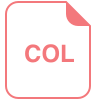
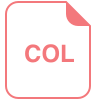
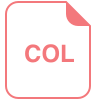
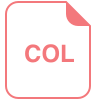
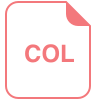
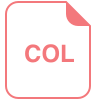
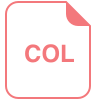
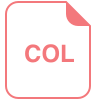
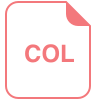
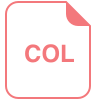

