怎么输出bytebuffer字符串里的内容
时间: 2024-02-23 08:01:03 浏览: 105
要输出 ByteBuffer 中的字符串内容,可以使用 ByteBuffer 的 `toString()` 方法将其转换为字符串,然后再用 `System.out.println()` 或其他输出方式输出。
下面是一个示例代码:
```java
ByteBuffer byteBuffer = ByteBuffer.allocate(1024); // 创建一个 ByteBuffer
byteBuffer.put("Hello World".getBytes()); // 往 ByteBuffer 中写入字符串
byteBuffer.flip(); // 切换为读模式
String content = new String(byteBuffer.array(), 0, byteBuffer.limit()); // 将 ByteBuffer 转换为字符串
System.out.println(content); // 输出字符串
```
这里使用 `getBytes()` 方法将字符串转换为字节数组,然后写入 ByteBuffer。在输出时,将 ByteBuffer 转换为字符串,然后输出。需要注意的是,要使用 `flip()` 方法切换为读模式,否则无法正确读取 ByteBuffer 中的内容。
相关问题
byteBuffer转 字符串
将 ByteBuffer 转换为字符串需要考虑字符编码的问题。以下是示例代码:
```java
import java.nio.charset.Charset;
import java.nio.ByteBuffer;
public class ByteBufferToString {
public static void main(String[] args) {
ByteBuffer buffer = ByteBuffer.wrap(new byte[] { 72, 101, 108, 108, 111 });
// 使用默认字符集解码
String str1 = Charset.defaultCharset().decode(buffer).toString();
System.out.println(str1); // 输出 Hello
// 使用指定字符集解码
Charset charset = Charset.forName("UTF-8");
buffer.rewind(); // 将 position 设为 0
String str2 = charset.decode(buffer).toString();
System.out.println(str2); // 输出 Hello
}
}
```
在示例代码中,首先创建了一个 ByteBuffer 对象,它包含了 5 个字节,表示字符串 "Hello"。然后使用 `Charset` 类提供的方法对 ByteBuffer 进行解码,得到字符串。第一个示例使用了默认字符集,而第二个示例指定了 UTF-8 编码。注意,在使用指定字符集解码之前,需要将 ByteBuffer 的 `position` 属性设置为 0,否则可能会出现解码错误。
ByteBuffer 转字符串
将 ByteBuffer 转换为字符串,可以使用 ByteBuffer 的 `Charset` 对象进行编码。以下是一个示例代码:
```java
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
public class ByteBufferToStringExample {
public static void main(String[] args) {
Charset charset = Charset.forName("UTF-8");
ByteBuffer byteBuffer = ByteBuffer.wrap("Hello, world!".getBytes(charset));
String str = charset.decode(byteBuffer).toString();
System.out.println(str);
}
}
```
在上面的代码中,我们首先使用 `Charset.forName()` 方法获取一个指定字符集的 `Charset` 对象,然后使用 `ByteBuffer.wrap()` 方法将字符串包装成 ByteBuffer 对象。接下来,我们使用 `Charset.decode()` 方法将 ByteBuffer 转换为字符串。最后,我们打印出字符串。
注意:在使用 `Charset.decode()` 方法之前,需要将 ByteBuffer 的 position 设置为 0,以便从 ByteBuffer 的开头开始解码。
阅读全文
相关推荐
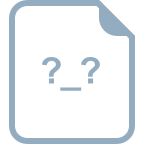
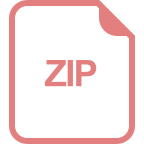
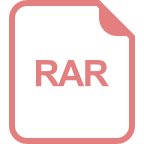



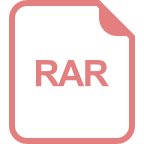
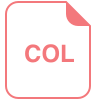








