题目是给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。请使用栈的java语言编写代码,并注明详细代码注释
时间: 2023-03-13 22:30:36 浏览: 85
// 使用栈来反转链表:
// Java 代码如下:
public ListNode reverseList(ListNode head) {
Stack<ListNode> stack = new Stack<>();
// 将 head 节点及其之后的节点全部入栈
ListNode currentNode = head;
while (currentNode != null) {
stack.push(currentNode);
currentNode = currentNode.next;
}
// 从栈中取出节点,并依次将其 next 指向前一个节点,
// 当取出 head 节点时,将其 next 设置为 null,完成链表反转
ListNode reverseHead = stack.pop();
ListNode tempNode = reverseHead;
while (!stack.isEmpty()) {
currentNode = stack.pop();
tempNode.next = currentNode;
tempNode = currentNode;
}
tempNode.next = null;
return reverseHead;
}
相关问题
题目是给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。请使用栈的java语言编写代码
public ListNode reverseListByStack(ListNode head) {
Stack<ListNode> stack = new Stack<>();
ListNode cur = head;
while (cur != null) {
stack.push(cur);
cur = cur.next;
}
ListNode newHead = stack.pop();
cur = newHead;
while (!stack.isEmpty()) {
cur.next = stack.pop();
cur = cur.next;
}
cur.next = null;
return newHead;
}
题目是给你单链表的头节点 head ,请你反转链表,并返回反转后的链表。请使用栈的java语言编写代码,每行代码都有注释。
//初始化栈
Stack<Node> stack = new Stack<Node>();
// 将链表节点依次压入栈中
Node cursor = head;
while(cursor != null){
stack.push(cursor);
cursor = cursor.next;
}
// 将栈中的节点反转放入链表中
head = stack.pop();
cursor = head;
while(!stack.isEmpty()){
cursor.next = stack.pop();
cursor = cursor.next;
}
// 将最后一个节点的next置空
cursor.next = null;
//返回反转后的链表
return head;
阅读全文
相关推荐
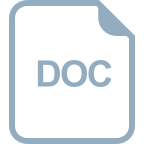
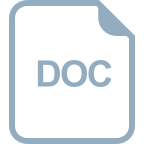
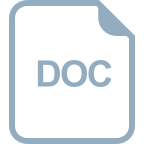













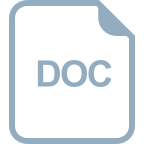