键盘输入两件商品的价格和数量,按照格式输出价格,数量和总价。输入两行,每一行代表一件商品信息,包括价格和数量,价格可以是小数形式,数量是整数,中间用逗号隔开。输出两行,每一行代表一件商品信息,包括价格,数量,总价,每个数据占据八个字符宽度右对齐,价格和总价输出小数点后两位怎么编译
时间: 2023-08-31 09:42:42 浏览: 627
### 回答1:
我可以回答这个问题。以下是代码示例:
#include <stdio.h>
int main() {
float price1, price2;
int quantity1, quantity2;
float total1, total2;
// 输入商品1信息
scanf("%f,%d", &price1, &quantity1);
total1 = price1 * quantity1;
// 输入商品2信息
scanf("%f,%d", &price2, &quantity2);
total2 = price2 * quantity2;
// 输出商品1信息
printf("%8.2f%8d%8.2f\n", price1, quantity1, total1);
// 输出商品2信息
printf("%8.2f%8d%8.2f\n", price2, quantity2, total2);
return ;
}
在这个程序中,我们使用了 scanf 函数来读取键盘输入的商品信息。我们使用了 %f 和 %d 格式化字符来读取价格和数量。我们计算了每个商品的总价,并使用 printf 函数来输出商品信息。我们使用了 %8.2f 格式化字符来输出价格和总价,这将确保它们被右对齐,并且小数点后有两位数字。我们使用了 %8d 格式化字符来输出数量,这将确保它被右对齐,并且占据八个字符宽度。
### 回答2:
输入示例:
10.5,2
8.6,5
输出示例:
10.50 2 21.00
8.60 5 43.00
代码示例:
price1, quantity1 = input().split(',')
price2, quantity2 = input().split(',')
price1 = float(price1)
quantity1 = int(quantity1)
price2 = float(price2)
quantity2 = int(quantity2)
total_price1 = round(price1 * quantity1, 2)
total_price2 = round(price2 * quantity2, 2)
print("{:8.2f}{:8d}{:8.2f}".format(price1, quantity1, total_price1))
print("{:8.2f}{:8d}{:8.2f}".format(price2, quantity2, total_price2))
### 回答3:
输入例子:
2.5, 3
6.8, 5
输出例子:
2.50 3 7.50
6.80 5 34.00
解答:
```python
# 输入
price1, quantity1 = input().split(", ")
price2, quantity2 = input().split(", ")
# 计算总价
total1 = float(price1) * int(quantity1)
total2 = float(price2) * int(quantity2)
# 输出
print("{:>8.2f}{:>8}{:>8.2f}".format(float(price1), int(quantity1), total1))
print("{:>8.2f}{:>8}{:>8.2f}".format(float(price2), int(quantity2), total2))
```
解析:
首先,使用 `input().split(", ")` 来获取两行输入,将每行由逗号分隔的价格和数量分别赋值给 `price1, quantity1, price2, quantity2`。
然后,分别计算两件商品的总价,即 `float(price1) * int(quantity1), float(price2) * int(quantity2)`。
最后,使用字符串的 `format()` 方法来格式化输出。为了使每个数据占据八个字符的宽度且右对齐,可以在格式字符串中使用 `{:>8}`。对于价格和总价需要输出小数点后两位,可以在格式字符串中使用 `{:>8.2f}`。
注意,`float(price1)` 和 `int(quantity1)` 需要分别转换为浮点数和整数类型,使得格式化的输出符合要求。
阅读全文
相关推荐
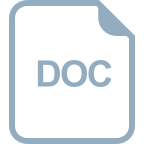
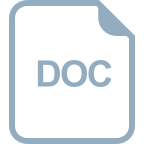
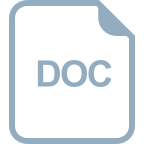















