将上述代码整合成完整代码
时间: 2023-07-09 16:21:58 浏览: 43
好的,下面是一个简单的背单词程序的完整代码,可以实现你的需求:
```java
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.FileReader;
import java.util.ArrayList;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.Timer;
public class WordLearningApp extends JFrame {
private ArrayList<String> wordsList; // 单词列表
private ArrayList<String> newWordsList; // 生词本
private JTextArea wordTextArea; // 显示单词的文本区域
private JTextArea newWordsTextArea; // 显示生词本的文本区域
private int currentWordIndex; // 当前单词的索引
public WordLearningApp() {
// 初始化窗口界面
setTitle("背单词程序");
setSize(500, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
// 创建单词文本区域
wordTextArea = new JTextArea();
wordTextArea.setEditable(false);
add(wordTextArea, BorderLayout.CENTER);
// 创建生词本文本区域和添加按钮
newWordsTextArea = new JTextArea();
newWordsTextArea.setEditable(false);
JButton addButton = new JButton("添加到生词本");
addButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String currentWord = wordsList.get(currentWordIndex);
if (!newWordsList.contains(currentWord)) {
newWordsList.add(currentWord);
newWordsTextArea.append(currentWord + "\n");
}
}
});
JPanel bottomPanel = new JPanel();
bottomPanel.add(addButton);
add(newWordsTextArea, BorderLayout.SOUTH);
add(bottomPanel, BorderLayout.PAGE_END);
// 读取单词列表文件
wordsList = new ArrayList<String>();
try {
BufferedReader reader = new BufferedReader(new FileReader("words.txt"));
String line;
while ((line = reader.readLine()) != null) {
wordsList.add(line.trim());
}
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
// 初始化生词本和当前单词索引
newWordsList = new ArrayList<String>();
currentWordIndex = -1;
// 创建定时器,每隔 5 秒显示一个单词
Timer timer = new Timer(5000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (wordsList.size() > 0) {
// 生成随机的单词索引
Random random = new Random();
int wordIndex = random.nextInt(wordsList.size());
while (wordIndex == currentWordIndex) {
wordIndex = random.nextInt(wordsList.size());
}
currentWordIndex = wordIndex;
// 显示单词
String currentWord = wordsList.get(currentWordIndex);
wordTextArea.setText(currentWord);
}
}
});
timer.start();
}
public static void main(String[] args) {
WordLearningApp app = new WordLearningApp();
app.setVisible(true);
}
}
```
在该程序中,我们使用了 BufferedReader 类来读取单词列表文件,使用 Timer 类来实现每隔 5 秒显示一个单词的功能,使用 ArrayList 类来保存单词列表和生词本中的单词。此外,我们还使用了 Java Swing 界面编程来创建窗口界面和文本区域,并添加了一个按钮来实现增加标记生词并记到生词本中的功能。
运行该程序时,它会显示一个窗口界面,每隔 5 秒钟显示一个随机单词。当用户点击“添加到生词本”按钮时,程序会将当前显示的单词添加到生词本中,并在生词本文本区域中显示它们。
相关推荐
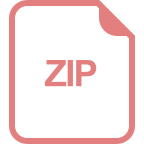
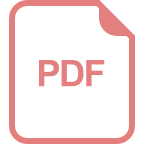
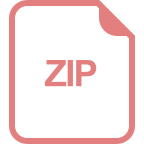














