java 传入文件的byte数组实现文件的分块下载
时间: 2024-04-10 15:28:14 浏览: 16
要实现基于字节数组的文件分块下载,你可以按照以下步骤进行操作:
1. 将文件读入字节数组中。
2. 根据需要分块的大小,确定每个分块的起始位置和结束位置。
3. 使用起始位置和结束位置,将相应的字节范围复制到一个新的字节数组中。
4. 将新的字节数组写入到输出流(比如`OutputStream`)中,发送给客户端。
5. 重复步骤3和4,直到读取完所有分块。
下面是一个简单的示例代码,用于实现基于字节数组的文件分块下载:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.OutputStream;
public class FileDownloader {
private static final int CHUNK_SIZE = 1024 * 1024; // 分块大小,这里设为1MB
public static void downloadFile(String filePath, OutputStream outputStream, int chunkIndex) throws IOException {
File file = new File(filePath);
long fileSize = file.length();
int totalChunks = (int) Math.ceil((double) fileSize / CHUNK_SIZE);
FileInputStream fileInputStream = new FileInputStream(file);
// 计算当前分块的起始位置和结束位置
int startByte = chunkIndex * CHUNK_SIZE;
int endByte = Math.min((chunkIndex + 1) * CHUNK_SIZE, (int) fileSize);
byte[] buffer = new byte[CHUNK_SIZE];
int bytesRead;
// 跳过前面的字节
long bytesSkipped = fileInputStream.skip(startByte);
if (bytesSkipped < startByte) {
// 处理跳过字节数不正确的情况
throw new IOException("Failed to skip bytes");
}
// 读取当前分块的字节到缓冲区
bytesRead = fileInputStream.read(buffer, 0, endByte - startByte);
while (bytesRead != -1) {
// 将字节数组写入到输出流
outputStream.write(buffer, 0, bytesRead);
outputStream.flush();
// 继续读取下一个分块的字节
bytesRead = fileInputStream.read(buffer, 0, CHUNK_SIZE);
}
fileInputStream.close();
}
}
```
你可以根据需要调用`downloadFile`方法来下载文件的各个分块。传递文件路径、输出流和分块索引作为参数,其中分块索引从0开始。注意根据需要适当处理异常和关闭相关资源。
请根据你的具体需求进行调整和扩展,例如添加错误处理、进度条显示等功能。
相关推荐
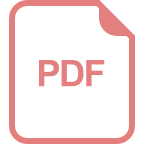
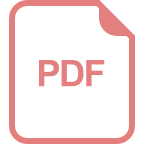
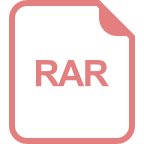














