stm32f103c8t6 VGA显示一串数字程序
时间: 2023-09-30 10:04:46 浏览: 155
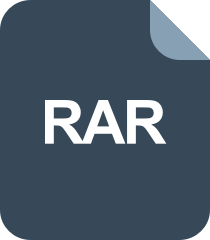
STM32驱动VGA显示器单色
以下是一个简单的示例代码,可以在 stm32f103c8t6 上使用 VGA 显示一串数字:
```c
#include "stm32f10x.h"
#define HSYNC_PIN GPIO_Pin_0
#define HSYNC_PORT GPIOA
#define VSYNC_PIN GPIO_Pin_1
#define VSYNC_PORT GPIOA
#define COLOR_PIN GPIO_Pin_2
#define COLOR_PORT GPIOA
#define HSYNC_TIM TIM2
#define HSYNC_TIM_RCC RCC_APB1Periph_TIM2
#define VGA_H_PIXELS 640
#define VGA_H_FRONT_PORCH 16
#define VGA_H_SYNC_PULSE 96
#define VGA_H_BACK_PORCH 48
#define VGA_H_TOTAL (VGA_H_PIXELS + VGA_H_FRONT_PORCH + VGA_H_SYNC_PULSE + VGA_H_BACK_PORCH)
#define VGA_V_PIXELS 480
#define VGA_V_FRONT_PORCH 10
#define VGA_V_SYNC_PULSE 2
#define VGA_V_BACK_PORCH 33
#define VGA_V_TOTAL (VGA_V_PIXELS + VGA_V_FRONT_PORCH + VGA_V_SYNC_PULSE + VGA_V_BACK_PORCH)
void VGA_Init(void);
void VGA_DrawPixel(uint16_t x, uint16_t y, uint16_t color);
void VGA_DrawChar(uint16_t x, uint16_t y, uint8_t c, uint16_t color);
void VGA_DrawString(uint16_t x, uint16_t y, char* str, uint16_t color);
void VGA_DrawNumber(uint16_t x, uint16_t y, uint32_t num, uint16_t color);
void VGA_HSyncHandler(void);
void VGA_VSyncHandler(void);
volatile uint16_t vga_frame_buffer[VGA_V_TOTAL][VGA_H_TOTAL];
int main(void)
{
SystemInit();
VGA_Init();
while (1)
{
VGA_DrawNumber(VGA_H_PIXELS / 2 - 40, VGA_V_PIXELS / 2 - 8, 12345678, 0xFFFF);
}
}
void VGA_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = HSYNC_PIN;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(HSYNC_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = VSYNC_PIN;
GPIO_Init(VSYNC_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = COLOR_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(COLOR_PORT, &GPIO_InitStructure);
RCC_APB1PeriphClockCmd(HSYNC_TIM_RCC, ENABLE);
TIM_TimeBaseStructure.TIM_Period = VGA_H_TOTAL - 1;
TIM_TimeBaseStructure.TIM_Prescaler = 72 - 1;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(HSYNC_TIM, &TIM_TimeBaseStructure);
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = VGA_H_SYNC_PULSE;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC1Init(HSYNC_TIM, &TIM_OCInitStructure);
TIM_ITConfig(HSYNC_TIM, TIM_IT_Update, ENABLE);
NVIC_InitStructure.NVIC_IRQChannel = TIM2_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
TIM_Cmd(HSYNC_TIM, ENABLE);
}
void VGA_DrawPixel(uint16_t x, uint16_t y, uint16_t color)
{
vga_frame_buffer[y][x] = color;
}
void VGA_DrawChar(uint16_t x, uint16_t y, uint8_t c, uint16_t color)
{
uint8_t i, j;
for (i = 0; i < 8; i++)
{
for (j = 0; j < 6; j++)
{
if ((Font6x8[c][j] >> i) & 0x01)
{
VGA_DrawPixel(x + j, y + i, color);
}
}
}
}
void VGA_DrawString(uint16_t x, uint16_t y, char* str, uint16_t color)
{
while (*str != '\0')
{
VGA_DrawChar(x, y, *str++, color);
x += 6;
}
}
void VGA_DrawNumber(uint16_t x, uint16_t y, uint32_t num, uint16_t color)
{
char str[10];
sprintf(str, "%lu", num);
VGA_DrawString(x, y, str, color);
}
void VGA_HSyncHandler(void)
{
static uint16_t h_count = 0;
if (h_count < VGA_H_PIXELS)
{
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 15));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 14));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 13));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 12));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 11));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 10));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 9));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 8));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 7));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 6));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 5));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 4));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 3));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 2));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)(vga_frame_buffer[VGA_V_TOTAL - 1][h_count] >> 1));
GPIO_WriteBit(COLOR_PORT, COLOR_PIN, (BitAction)vga_frame_buffer[VGA_V_TOTAL - 1][h_count]);
}
h_count++;
if (h_count >= VGA_H_TOTAL)
{
h_count = 0;
TIM_ClearITPendingBit(HSYNC_TIM, TIM_IT_Update);
VGA_VSyncHandler();
}
}
void VGA_VSyncHandler(void)
{
static uint16_t v_count = 0;
if (v_count < VGA_V_PIXELS)
{
v_count++;
}
if (v_count == VGA_V_FRONT_PORCH)
{
GPIO_WriteBit(VSYNC_PORT, VSYNC_PIN, Bit_RESET);
}
if (v_count == VGA_V_FRONT_PORCH + VGA_V_SYNC_PULSE)
{
GPIO_WriteBit(HSYNC_PORT, HSYNC_PIN, Bit_RESET);
}
if (v_count == VGA_V_FRONT_PORCH + VGA_V_SYNC_PULSE + VGA_V_BACK_PORCH)
{
GPIO_WriteBit(HSYNC_PORT, HSYNC_PIN, Bit_SET);
}
if (v_count == VGA_V_TOTAL - 1)
{
v_count = 0;
GPIO_WriteBit(VSYNC_PORT, VSYNC_PIN, Bit_SET);
}
}
void TIM2_IRQHandler(void)
{
if (TIM_GetITStatus(HSYNC_TIM, TIM_IT_Update) != RESET)
{
TIM_ClearITPendingBit(HSYNC_TIM, TIM_IT_Update);
VGA_HSyncHandler();
}
}
```
注意,此代码仅显示数字,您需要在代码中添加更多功能来实现您的需求。此外,您还需要将 STM32F103C8T6 连接到 VGA 显示器,以便显示输出。
阅读全文
相关推荐
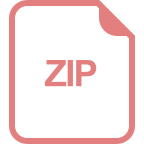





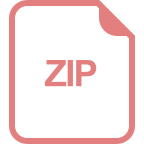
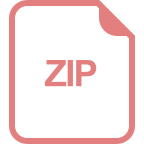
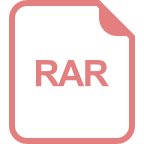

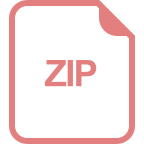
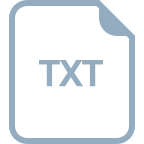
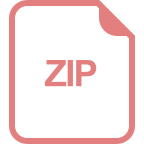