编写程序,实现对数据库studentInfo中的表student进行插入操作。要求使用预处理语句对象PreparedStatament完成以下数据的插入
时间: 2024-01-21 14:17:07 浏览: 73
以下是一个Java程序示例,演示如何使用PreparedStatement对象向studentInfo数据库中的student表插入数据:
```java
import java.sql.*;
public class InsertData {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/studentInfo";
String username = "root";
String password = "password";
Connection conn = null;
PreparedStatement pstmt = null;
try {
// 创建数据库连接
conn = DriverManager.getConnection(url, username, password);
// 构造SQL语句,使用占位符 ? 代替实际的参数值
String sql = "INSERT INTO student (id, name, age, gender) VALUES (?, ?, ?, ?)";
// 创建PreparedStatement对象,并设置占位符对应的参数值
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, 1001); // 第一个占位符对应id列,设置值为1001
pstmt.setString(2, "张三"); // 第二个占位符对应name列,设置值为"张三"
pstmt.setInt(3, 20); // 第三个占位符对应age列,设置值为20
pstmt.setString(4, "男"); // 第四个占位符对应gender列,设置值为"男"
// 执行插入操作
pstmt.executeUpdate();
System.out.println("数据插入成功!");
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭PreparedStatement对象和数据库连接
try {
if (pstmt != null) {
pstmt.close();
}
if (conn != null) {
conn.close();
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
注意,上述代码中使用了占位符 ? 代替实际的参数值,并使用PreparedStatement对象的setXxx()方法设置占位符对应的参数值,这样可以避免SQL注入攻击。同时,还需注意在finally块中关闭PreparedStatement对象和数据库连接,以释放资源。
阅读全文
相关推荐
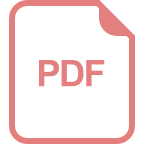









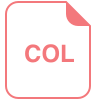
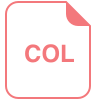
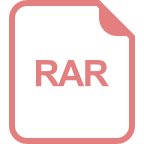
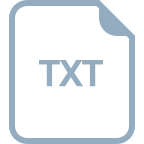
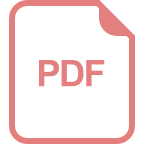
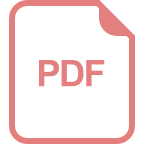
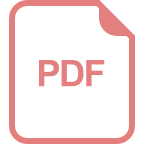