java Web编写程序,实现对数据库StudentInfo中标student进行插入操作。要求使用预处理语句对象PrepareStatement完成以下数据的插入.要求使用预处理语句对象PrepareStatement完成数据的插入。
时间: 2024-01-21 13:18:18 浏览: 24
下面是一个示例代码,可以实现对数据库中的StudentInfo表进行插入操作:
```java
import java.sql.*;
public class StudentInsert {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement pstmt = null;
try {
// 1. 加载数据库驱动
Class.forName("com.mysql.jdbc.Driver");
// 2. 建立数据库连接
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/StudentDB", "root", "password");
// 3. 创建PreparedStatement对象
String sql = "INSERT INTO StudentInfo (name, age, gender) VALUES (?, ?, ?)";
pstmt = conn.prepareStatement(sql);
// 4. 设置参数
pstmt.setString(1, "张三");
pstmt.setInt(2, 20);
pstmt.setString(3, "男");
// 5. 执行插入操作
int rows = pstmt.executeUpdate();
System.out.println(rows + " 行记录被插入");
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 6. 关闭数据库连接和PreparedStatement对象
try {
if (pstmt != null) pstmt.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
上述代码中,首先加载了MySQL数据库驱动,然后建立了一个与StudentDB数据库的连接。接着,使用PrepareStatement对象来创建一个插入SQL语句,并且使用setXXX()方法来设置每个参数的值。最后,调用executeUpdate()方法来执行插入操作,并输出结果。
需要注意的是,在实际编程中,需要根据不同的情况来设置参数的值,比如从用户输入中获取参数值,或者从其他对象中获取参数值。同时,还需要在程序中处理异常情况,如数据库连接失败、SQL语句执行失败等。
相关推荐
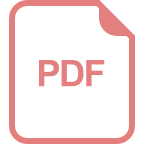
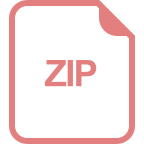
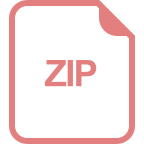














