组内同学分别使用Calendar和Date类取得当前时间,并以以下格式输出: 2022-10-18 12:00:02 星期二 请
时间: 2024-10-20 12:19:26 浏览: 22
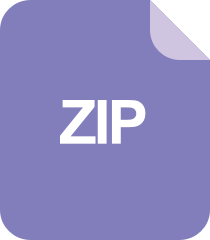
react-native-calendarview-datepicker::herb: React-native-calendar-date-picker 组件
在Java中,你可以使用`java.util.Calendar`和`java.util.Date`这两个类来获取当前时间和日期。以下是使用这两个类分别获取并按照指定格式输出的一个示例:
首先,使用`Calendar`类:
```java
import java.util.Calendar;
public class Main {
public static void main(String[] args) {
// 获取当前时间
Calendar calendar = Calendar.getInstance();
// 设置日期格式(年-月-日)
int year = calendar.get(Calendar.YEAR);
int month = calendar.get(Calendar.MONTH) + 1; // 因为get返回的是0-based index
int day = calendar.get(Calendar.DAY_OF_MONTH);
// 设置时间格式(小时:分钟:秒)
int hour = calendar.get(Calendar.HOUR_OF_DAY);
int minute = calendar.get(Calendar.MINUTE);
int second = calendar.get(Calendar.SECOND);
// 格式化并输出
String formattedDate = String.format("%d-%02d-%02d %02d:%02d:%02d", year, month, day, hour, minute, second);
System.out.println(formattedDate);
// 输出星期几,这里需要额外处理,因为Calendar没有直接提供对应的字符串
String dayOfWeekStr = getDayOfWeekString(calendar);
System.out.println(dayOfWeekStr);
}
// 自定义方法将Calendar的星期索引转换为英文星期名称
private static String getDayOfWeekString(Calendar calendar) {
switch (calendar.get(Calendar.DAY_OF_WEEK)) {
case Calendar.SUNDAY:
return "星期天";
case Calendar.MONDAY:
return "星期一";
// ...添加其他case到周日
default:
return "未知";
}
}
}
```
然后,使用`java.util.Date`类(但请注意`Date`已经不再推荐使用,因为它不是线程安全的,建议升级到`java.time.LocalDate`和`java.time.LocalDateTime`等更现代的时间类):
```java
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
// 获取当前时间
Date currentDate = new Date();
// 使用SimpleDateFormat设置日期和时间格式
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String formattedDateTime = sdf.format(currentDate);
System.out.println(formattedDateTime);
// 获取星期几,使用SimpleDateFormat辅助
SimpleDateFormat dayOfWeekSdf = new SimpleDateFormat("EEEE");
String dayOfWeek = dayOfWeekSdf.format(currentDate);
System.out.println(dayOfWeek);
}
}
```
注意:`Date`和`Calendar`的示例都假设了你有一个可用的日历系统。如果你在特定环境中遇到问题,比如没有网络连接无法获取正确的当前日期,这些代码可能不会工作。
阅读全文
相关推荐
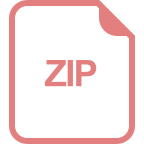
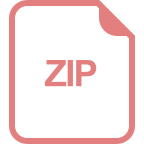















