代码训练速度慢,正确率低
时间: 2024-10-26 09:03:22 浏览: 27
根据您提供的代码,以下是一些可能的原因及改进建议,以提高训练速度和模型性能:
### 1. **优化学习率**
- 当前使用的学习率为 `0.0001`,可以尝试调整学习率,例如从 `0.001` 开始,逐步减小到 `0.0001` 或更小。
```python
model.compile(optimizer=Adam(learning_rate=0.001), loss='sparse_categorical_crossentropy', metrics=['accuracy'])
```
### 2. **增加数据增强**
- 当前的数据增强已经比较全面,但可以考虑进一步增加数据增强的强度或种类,例如添加颜色抖动(color jittering)等。
```python
datagen = ImageDataGenerator(
rotation_range=30,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
vertical_flip=True,
brightness_range=[0.5, 1.5],
channel_shift_range=0.2,
fill_mode='nearest'
)
```
### 3. **使用预训练权重**
- 当前模型使用的是无预训练权重的 ResNet50,这可能导致模型需要更多时间来学习特征。建议使用预训练权重(如 ImageNet),这样可以加速收敛并提高准确率。
```python
def create_resnet_model(input_shape=(128, 128, 3)):
base_model = ResNet50(weights='imagenet', include_top=False, input_shape=input_shape)
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(256, activation='relu')(x)
x = Dropout(0.5)(x)
predictions = Dense(4, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=predictions)
return model
```
### 4. **减少过拟合**
- 增加正则化项,例如 L2 正则化,可以在模型中加入 `kernel_regularizer`。
```python
from tensorflow.keras.regularizers import l2
def create_resnet_model(input_shape=(128, 128, 3)):
base_model = ResNet50(weights='imagenet', include_top=False, input_shape=input_shape)
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(256, activation='relu', kernel_regularizer=l2(0.01))(x)
x = Dropout(0.5)(x)
predictions = Dense(4, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=predictions)
return model
```
### 5. **调整模型结构**
- 可以尝试不同的网络结构,例如增加或减少层数,调整层的大小等。
```python
def create_resnet_model(input_shape=(128, 128, 3)):
base_model = ResNet50(weights='imagenet', include_top=False, input_shape=input_shape)
x = base_model.output
x = GlobalAveragePooling2D()(x)
x = Dense(512, activation='relu')(x)
x = Dropout(0.5)(x)
x = Dense(128, activation='relu')(x)
x = Dropout(0.5)(x)
predictions = Dense(4, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=predictions)
return model
```
### 6. **早停法(Early Stopping)**
- 使用早停法可以防止过度训练,同时节省计算资源。
```python
from tensorflow.keras.callbacks import EarlyStopping
early_stopping = EarlyStopping(monitor='val_loss', patience=10, restore_best_weights=True)
history = model.fit(datagen.flow(X_train, y_train, batch_size=32),
epochs=50,
validation_data=(X_val, y_val),
callbacks=[early_stopping])
```
### 7. **检查数据质量**
- 确保训练数据的质量,包括图像的清晰度、标注的准确性等。如果数据质量不高,模型的表现也会受到影响。
通过上述改进,您可以尝试提高模型的训练速度和准确率。希望这些建议对您有所帮助!
阅读全文
相关推荐
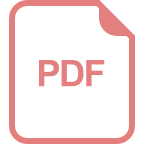
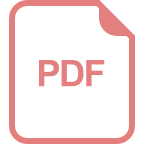
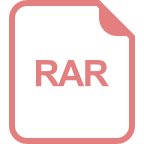

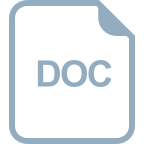
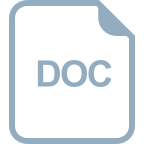
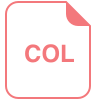
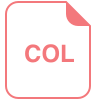
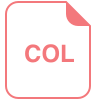
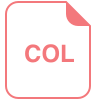
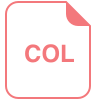
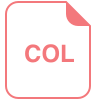
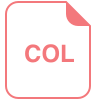
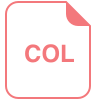
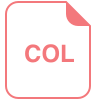
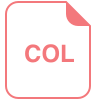
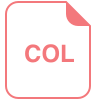
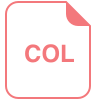
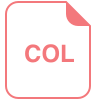