写一个带图像的贪吃蛇C++
时间: 2024-01-06 18:03:45 浏览: 22
以下是一个基于C++的贪吃蛇游戏,包含了图像和基本游戏操作。请注意,由于涉及到图像操作,需要使用相应的图像库,如OpenCV。
```c++
#include <iostream>
#include <vector>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
// 定义方向枚举
enum Direction {
UP,
DOWN,
LEFT,
RIGHT
};
// 定义游戏参数
const int WIDTH = 640;
const int HEIGHT = 480;
const int BLOCK_SIZE = 20;
// 定义贪吃蛇类
class Snake {
public:
// 构造函数
Snake(int x, int y) {
body.push_back(Point(x, y));
direction = RIGHT;
}
// 获取头部位置
Point getHead() {
return body.front();
}
// 获取尾部位置
Point getTail() {
return body.back();
}
// 获取身体长度
int getLength() {
return body.size();
}
// 移动
void move() {
// 添加新头部
Point newHead = getHead();
switch(direction) {
case UP:
newHead.y -= BLOCK_SIZE;
break;
case DOWN:
newHead.y += BLOCK_SIZE;
break;
case LEFT:
newHead.x -= BLOCK_SIZE;
break;
case RIGHT:
newHead.x += BLOCK_SIZE;
break;
}
body.insert(body.begin(), newHead);
// 删除尾部
body.pop_back();
}
// 改变方向
void changeDirection(Direction dir) {
if (getLength() > 1 && (direction + dir) % 2 == 0) {
// 不允许反向运动
return;
}
direction = dir;
}
// 增加长度
void grow() {
body.push_back(getTail());
}
// 判断是否碰撞
bool collide() {
Point head = getHead();
// 碰到边界
if (head.x < 0 || head.x >= WIDTH || head.y < 0 || head.y >= HEIGHT) {
return true;
}
// 碰到自己身体
for (int i = 1; i < getLength(); i++) {
if (head == body[i]) {
return true;
}
}
return false;
}
private:
vector<Point> body; // 身体坐标
Direction direction; // 运动方向
};
// 定义食物类
class Food {
public:
// 构造函数
Food() {
generate();
}
// 获取位置
Point getPosition() {
return position;
}
// 绘制
void draw(Mat& img) {
Scalar color(0, 255, 0); // 绿色
rectangle(img, position, position + Point(BLOCK_SIZE, BLOCK_SIZE), color, -1);
}
// 生成新位置
void generate() {
int x = rand() % (WIDTH / BLOCK_SIZE) * BLOCK_SIZE;
int y = rand() % (HEIGHT / BLOCK_SIZE) * BLOCK_SIZE;
position = Point(x, y);
}
private:
Point position; // 坐标
};
int main() {
// 创建窗口
namedWindow("Snake", WINDOW_AUTOSIZE);
// 创建画布
Mat canvas = Mat::zeros(HEIGHT, WIDTH, CV_8UC3);
// 创建贪吃蛇和食物
Snake snake(WIDTH / 2, HEIGHT / 2);
Food food;
// 设置随机种子
srand(time(NULL));
// 游戏循环
while (true) {
// 绘制背景
canvas.setTo(Scalar(0, 0, 0)); // 黑色
// 绘制贪吃蛇
Scalar color(255, 255, 255); // 白色
for (auto& p : snake.getBody()) {
rectangle(canvas, p, p + Point(BLOCK_SIZE, BLOCK_SIZE), color, -1);
}
// 绘制食物
food.draw(canvas);
// 显示画布
imshow("Snake", canvas);
// 等待输入
int key = waitKey(100);
// 处理输入
switch (key) {
case 'w':
snake.changeDirection(UP);
break;
case 's':
snake.changeDirection(DOWN);
break;
case 'a':
snake.changeDirection(LEFT);
break;
case 'd':
snake.changeDirection(RIGHT);
break;
case 'q':
return 0;
}
// 移动贪吃蛇
snake.move();
// 判断是否碰撞
if (snake.collide()) {
cout << "Game over!" << endl;
return 0;
}
// 检查是否吃到食物
if (snake.getHead() == food.getPosition()) {
snake.grow();
food.generate();
}
}
return 0;
}
```
上述代码使用OpenCV库进行图像绘制,并且包含了基本的游戏操作和碰撞检测。运行后,按WASD键控制贪吃蛇的运动方向,Q键退出游戏。
相关推荐








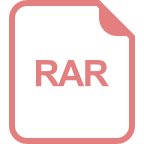
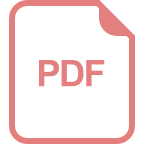
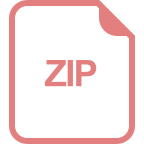