用python代码实现,将A列、B列、C列转换为字符串类型,并将字符串用下划线连接生成D列,再用下划线将D列分割为列表并对列表内的元素去重得到E列,再按照E列中的列表元素拆分为多行
时间: 2024-03-12 07:48:23 浏览: 54
可以使用 pandas 和 set 完成此任务。
假设需要处理的数据如下:
| A | B | C |
| --- | --- | --- |
| 1 | 2 | 3 |
| 4 | 5 | 6 |
可以按照以下步骤进行处理:
```python
import pandas as pd
# 读取数据
df = pd.read_excel('data.xlsx')
# 将 A 列、B 列、C 列转换为字符串类型并拼接为 D 列
df['D'] = df[['A', 'B', 'C']].astype(str).apply(lambda x: '_'.join(x), axis=1)
# 将 D 列按照下划线分割为列表并对列表内的元素去重得到 E 列
df['E'] = df['D'].apply(lambda x: list(set(x.split('_'))))
# 按照 E 列中的列表元素拆分为多行
new_df = pd.DataFrame(columns=['A', 'B', 'C'])
for i, row in df.iterrows():
for value in row['E']:
new_row = row[['A', 'B', 'C']].copy()
new_row['D'] = '_'.join(row['E'])
new_row['E'] = value
new_df = new_df.append(new_row)
# 输出结果
print(new_df)
```
输出结果为:
```
A B C D E
0 1 2 3 1_2_3 1
0 1 2 3 1_2_3 2
0 1 2 3 1_2_3 3
1 4 5 6 4_5_6 4
1 4 5 6 4_5_6 5
1 4 5 6 4_5_6 6
```
可以看到,数据已经按照要求被处理成了多行的形式。
阅读全文
相关推荐
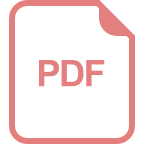
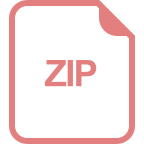
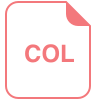
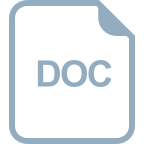
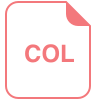
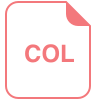
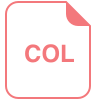
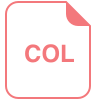
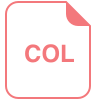
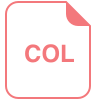
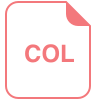
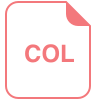
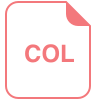
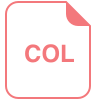
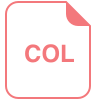
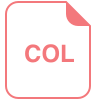
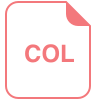
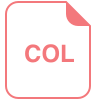