实现线性表顺序存储(数据类型为int类型或char类型), 1创建文件exp1-1-1.cpp随机输入5个字符(或对于给定的5个字符a,b,c,d,e),创建顺序表;求长度; 获取第3个元素的值;在第4个元素前插入元素g,删除第5个元素,销毁创建的线性表。
时间: 2024-09-19 13:04:16 浏览: 58
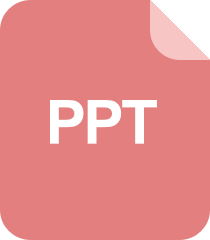
数据结构(Java版)线性表1(顺序表).ppt
要实现线性表顺序存储,你可以按照以下步骤进行:
1. 定义一个结构体表示节点,包含数据域和指向下一个节点的指针[^1]:
```cpp
// SqList.h
struct Node {
int data; // 或 char data (取决于数据类型)
Node* next;
};
```
2. 创建顺序表类,包括构造函数、获取长度的方法、访问特定元素的方法以及插入和删除元素的方法:
```cpp
class SeqList {
private:
Node* head;
int length;
public:
SeqList(int capacity = 5); // 构造函数,可以预设容量
~SeqList(); // 析构函数,释放内存
void insert(int index, int value); // 在指定索引处插入元素
void deleteElement(int index); // 删除指定索引的元素
int getLength(); // 返回序列表长度
int getElement(int index); // 返回指定索引的元素
};
// 实现相应方法...
```
3. 实现插入、删除和获取元素的具体逻辑:
```cpp
void SeqList::insert(int index, int value) {
if (index < 0 || index > length) {
throw std::out_of_range("Index out of range");
}
Node* newNode = new Node{value, nullptr}; // 新节点
if (index == 0) {
newNode->next = head;
head = newNode;
} else {
Node* current = head;
for (int i = 0; i < index - 1; ++i) {
current = current->next;
}
newNode->next = current->next;
current->next = newNode;
}
length++;
}
void SeqList::deleteElement(int index) {
if (index < 0 || index >= length) {
throw std::out_of_range("Index out of range");
}
if (index == 0) {
head = head->next;
} else {
Node* current = head;
for (int i = 0; i < index - 1; ++i) {
current = current->next;
}
current->next = current->next->next;
}
delete head->next;
length--;
}
int SeqList::getElement(int index) {
if (index < 0 || index >= length) {
throw std::out_of_range("Index out of range");
}
Node* current = head;
for (int i = 0; i < index; ++i) {
current = current->next;
}
return current->data;
}
```
4. 主程序`exp2_1.cpp`中调用这些方法来完成所需操作[^2]:
```cpp
#include "SqList.h"
int main() {
SeqList seqList(5); // 初始化顺序表
// 随机输入或给定数据
// seqList.insert(index, value);
// seqList.deleteElement(index);
// int element = seqList.getLength();
// int thirdElement = seqList.getElement(2);
// 执行上述操作后...
return 0;
}
```
阅读全文
相关推荐
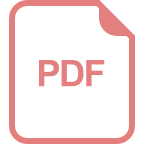















