实现线性表顺序存储(数据类型为int类型或char类型), 1创建文件exp1-1-1.cpp随机输入5个字符(或对于给定的5个字符a,b,c,d,e),创建顺序表;求长度; 获取第3个元素的值;在第4个元素前插入元素g,删除第5个元素,销毁创建的线性表,显示完整代码
时间: 2024-09-19 13:04:21 浏览: 58
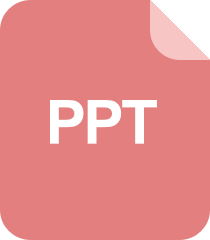
表ADT-1 表-顺序存储结构
为了实现线性表顺序存储,你可以按照以下步骤编写`exp1-1-1.cpp`代码:
首先,我们需要定义一个简单的结构体表示单个节点,接着创建一个类`SqList`来管理顺序表。这里以`int`类型为例,因为题目未明确指定字符类型,我们可以先假设为`int`。
1. 定义一个`Node`结构体,用于存储元素和指向下一个节点的指针:
```cpp
// SqList.h (包含头文件)
struct Node {
int data;
Node* next;
};
```
2. 在`SqList.h`中继续添加顺序表类的接口,包括构造函数、析构函数以及基本操作的声明:
```cpp
class SqList {
public:
// 构造函数
SqList();
// 析构函数
~SqList();
// 添加元素到尾部
void insert(int value);
// 求长度
int length();
// 获取第n个元素
int get(int index);
// 在指定位置插入元素
void insertAt(int index, int value);
// 删除指定索引的元素
void deleteAt(int index);
private:
Node* head; // 表头指针
int size; // 当前元素数量
};
```
3. 实现上述接口的方法:
```cpp
// SqList.cpp (实现文件)
#include "SqList.h"
// 构造函数
SqList::SqList() : head(nullptr), size(0) {}
// 析构函数
SqList::~SqList() {
while (head) {
Node* temp = head;
head = head->next;
delete temp;
}
}
// 插入元素
void SqList::insert(int value) {
Node* newNode = new Node{value, nullptr};
if (!head) {
head = newNode;
} else {
Node* current = head;
while (current->next) {
current = current->next;
}
current->next = newNode;
}
size++;
}
// 求长度
int SqList::length() {
return size;
}
// 获取第n个元素
int SqList::get(int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
Node* current = head;
for (int i = 0; i < index; ++i) {
current = current->next;
}
return current->data;
}
// 在指定位置插入元素
void SqList::insertAt(int index, int value) {
if (index < 0 || index > size) {
throw std::out_of_range("Index out of range");
}
Node* newNode = new Node{value, nullptr};
if (index == 0) {
newNode->next = head;
head = newNode;
} else {
Node* previous = head;
for (int i = 0; i < index - 1; ++i) {
previous = previous->next;
}
newNode->next = previous->next;
previous->next = newNode;
}
size++;
}
// 删除指定索引的元素
void SqList::deleteAt(int index) {
if (index < 0 || index >= size) {
throw std::out_of_range("Index out of range");
}
if (index == 0) {
Node* temp = head;
head = head->next;
delete temp;
} else {
Node* previous = head;
for (int i = 0; i < index - 1; ++i) {
previous = previous->next;
}
previous->next = previous->next->next;
}
size--;
}
```
现在你可以在`exp2_1.cpp`中这样调用这些方法:
```cpp
// exp2_1.cpp
#include "SqList.h"
#include <iostream>
#include <cstdlib> // 用于rand和srand
int main() {
SqList myList;
srand(time(0)); // 初始化随机数种子
for (int i = 0; i < 5; ++i) {
int randomValue = rand() % 100; // 随机生成0-99的整数
myList.insert(randomValue); // 插入元素
std::cout << "Inserted: " << randomValue << std::endl;
}
std::cout << "Length: " << myList.length() << std::endl;
try {
std::cout << "Element at index 3: " << myList.get(3) << std::endl;
myList.insertAt(3, 'g'); // 在第四个元素前插入字符g
std::cout << "After inserting 'g': ";
myList.print(); // 自定义打印功能,假设它已存在
} catch (const std::out_of_range& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
try {
myList.deleteAt(4); // 删除第五个元素
std::cout << "After deleting: ";
myList.print();
} catch (const std::out_of_range& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
记得为`SqList`类添加一个`print()`方法,以便在控制台打印整个顺序表的内容。完成后,运行`exp2_1.cpp`即可看到所需的功能。
阅读全文
相关推荐
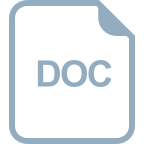
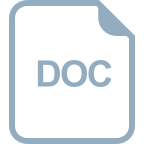

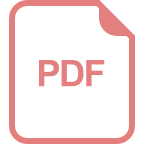
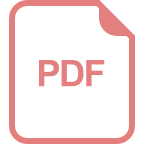
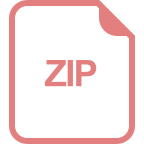