CountDownLatch加线程池
时间: 2023-08-18 22:14:46 浏览: 91
您好!要使用 `CountDownLatch` 与线程池配合使用,可以按照以下步骤进行操作:
1. 首先,创建一个 `CountDownLatch` 对象,并设置初始计数值。计数值表示等待的线程数量。
2. 创建一个线程池,可以使用 `ExecutorService` 接口的实现类,比如 `ThreadPoolExecutor`。
3. 将要执行的任务封装成 `Runnable` 对象,并提交给线程池执行。
4. 在任务中,当任务执行完毕之后,调用 `CountDownLatch` 的 `countDown()` 方法来减少计数值。
5. 在主线程中,调用 `CountDownLatch` 的 `await()` 方法来等待所有任务执行完毕。当计数值变为0时,主线程将被唤醒。
以下是一个简单的示例代码:
```java
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class CountDownLatchExample {
public static void main(String[] args) {
int threadCount = 5;
CountDownLatch latch = new CountDownLatch(threadCount);
ExecutorService executor = Executors.newFixedThreadPool(threadCount);
for (int i = 0; i < threadCount; i++) {
executor.execute(new WorkerRunnable(latch, "Task " + i));
}
try {
latch.await(); // 等待所有任务执行完毕
System.out.println("All tasks have finished!");
} catch (InterruptedException e) {
e.printStackTrace();
}
executor.shutdown();
}
}
class WorkerRunnable implements Runnable {
private final CountDownLatch latch;
private final String taskName;
public WorkerRunnable(CountDownLatch latch, String taskName) {
this.latch = latch;
this.taskName = taskName;
}
@Override
public void run() {
try {
System.out.println(taskName + " is running");
// 模拟任务执行时间
Thread.sleep(1000);
System.out.println(taskName + " has finished");
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
latch.countDown(); // 任务执行完毕,计数值减少
}
}
}
```
在这个示例中,我们创建了一个包含 5 个线程的线程池,并使用 `CountDownLatch` 设置等待的线程数量为 5。每个任务执行时,都会打印相应的任务名称,并模拟任务执行时间为 1 秒。当所有任务执行完毕后,主线程将被唤醒,并打印 "All tasks have finished!"。
希望能对您有所帮助!如果有任何疑问,请随时提问。
阅读全文
相关推荐
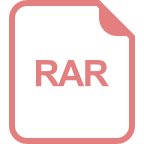
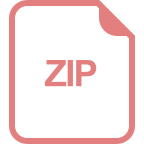











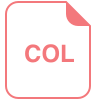



