使用CountDownLatch怎么在使用完一次线程池,执行完以后执行一些操作完成以后在执行下一个CountDownLatch操作的线程池
时间: 2023-08-14 10:05:04 浏览: 79
使用 `CountDownLatch` 可以在使用完一个线程池后执行一些操作,待这些操作完成后再执行下一个 `CountDownLatch` 操作的线程池。下面是一个示例代码,演示了如何使用 `CountDownLatch` 来实现这个功能:
```java
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) throws InterruptedException {
// 创建两个CountDownLatch,一个用于控制第一个线程池的操作,一个用于控制第二个线程池的操作
CountDownLatch latch1 = new CountDownLatch(1);
CountDownLatch latch2 = new CountDownLatch(1);
// 第一个线程池
ExecutorService executor1 = Executors.newFixedThreadPool(5);
executor1.submit(() -> {
try {
// 执行第一个线程池的操作
System.out.println("Executing tasks in the first thread pool");
Thread.sleep(1000); // 模拟任务执行
System.out.println("Tasks in the first thread pool completed");
// 执行一些其他操作
System.out.println("Performing additional tasks");
latch1.countDown(); // 当前线程池任务执行完毕,释放latch1
} catch (InterruptedException e) {
e.printStackTrace();
}
});
// 第二个线程池
ExecutorService executor2 = Executors.newFixedThreadPool(5);
executor2.submit(() -> {
try {
latch1.await(); // 等待latch1释放
// 执行第二个线程池的操作
System.out.println("Executing tasks in the second thread pool");
Thread.sleep(1000); // 模拟任务执行
System.out.println("Tasks in the second thread pool completed");
latch2.countDown(); // 当前线程池任务执行完毕,释放latch2
} catch (InterruptedException e) {
e.printStackTrace();
}
});
// 等待第二个线程池执行完毕
latch2.await();
System.out.println("All tasks completed");
// 关闭线程池
executor1.shutdown();
executor2.shutdown();
}
}
```
在上述示例中,首先创建了两个 `CountDownLatch` 对象:`latch1` 和 `latch2`。然后创建了两个线程池 `executor1` 和 `executor2`,分别用于执行第一个和第二个任务。
在第一个线程池中的任务执行完成后,执行一些其他操作,然后通过 `latch1.countDown()` 释放 `latch1`。
在第二个线程池中的任务中,通过 `latch1.await()` 等待 `latch1` 的释放。当 `latch1` 被释放后,第二个线程池中的任务开始执行。
最后,通过 `latch2.await()` 等待 `latch2` 的释放,以确保第二个线程池中的任务全部执行完毕。然后输出 "All tasks completed" 表示整个任务流程执行完毕。
需要注意的是,在实际使用中,需要根据具体情况设置合适的线程池大小、任务内容和等待时间等参数。
阅读全文
相关推荐
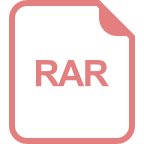
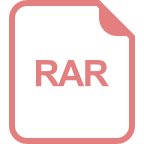

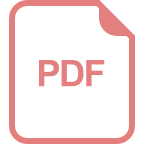













