python实现逻辑回归算法
时间: 2023-11-18 15:53:27 浏览: 45
好的,以下是Python实现逻辑回归算法的步骤:
1. 导入必要的库,如numpy、pandas等。
2. 读取数据集,将数据集分为训练集和测试集。
3. 对数据进行预处理,如缺失值填充、特征标准化等。
4. 定义逻辑回归模型,包括模型参数和损失函数。
5. 使用梯度下降法或其他优化算法来最小化损失函数,得到模型参数。
6. 使用训练好的模型对测试集进行预测,并计算预测准确率。
下面是一个简单的示例代码:
```python
import numpy as np
import pandas as pd
# 读取数据集
data = pd.read_csv('data.csv')
train_data = data.iloc[:80, :]
test_data = data.iloc[80:, :]
# 数据预处理
train_x = train_data.iloc[:, :-1]
train_y = train_data.iloc[:, -1]
test_x = test_data.iloc[:, :-1]
test_y = test_data.iloc[:, -1]
# 定义逻辑回归模型
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def logistic_regression(x, y, alpha, num_iters):
m, n = x.shape
theta = np.zeros(n)
for i in range(num_iters):
z = np.dot(x, theta)
h = sigmoid(z)
gradient = np.dot(x.T, (h - y)) / m
theta -= alpha * gradient
return theta
# 训练模型
theta = logistic_regression(train_x, train_y, alpha=0.01, num_iters=1000)
# 预测测试集
pred_y = sigmoid(np.dot(test_x, theta))
pred_y[pred_y >= 0.5] = 1
pred_y[pred_y < 0.5] = 0
accuracy = np.mean(pred_y == test_y)
print('Accuracy:', accuracy)
```
相关推荐
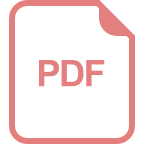
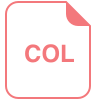
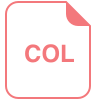
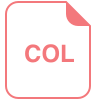
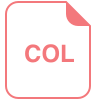
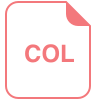









