在c语言中,对序列进行快速排序
时间: 2024-10-18 17:22:50 浏览: 13
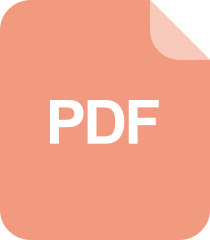
C语言对磁盘文件进行快速排序简单实例
在C语言中,快速排序是一种常用的高效排序算法,它基于分治策略。以下是快速排序的基本步骤:
1. **选择基准** (pivot):通常选取数组的第一个元素作为基准。
2. **分区操作** (Partitioning):
- 将数组划分为两部分:一部分所有元素都小于基准,另一部分所有元素都大于等于基准。
- 使用两个指针(一个开始于数组左侧,一个结束于右侧),通过比较元素值找到正确的位置交换,保证右侧的所有元素都比基准大,左侧的所有元素都比基准小。
3. **递归** (Recursion):
- 对划分后的两个子数组分别进行同样的快速排序过程,直到子数组只有一个元素或者为空,排序完成。
4. **合并结果** (Combining Results):当所有子数组排序后,将它们按原顺序连接起来就得到最终有序数组。
下面是简单的快速排序的C语言代码示例:
```c
#include <stdio.h>
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
int partition(int arr[], int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) high--;
if (low < high)
swap(&arr[low++], &arr[high]);
while (low < high && arr[low] <= pivot) low++;
if (low < high)
swap(&arr[low], &arr[high--]);
}
swap(&arr[low], &arr[high]);
return low;
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
// 测试函数
void printArray(int arr[], int size) {
int i;
for (i=0; i < size; i++)
printf("%d ", arr[i]);
printf("\n");
}
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr)/sizeof(arr[0]);
quickSort(arr, 0, n-1);
printf("Sorted array: \n");
printArray(arr, n);
return 0;
}
```
阅读全文
相关推荐
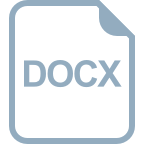
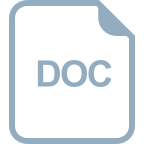















