顺序表存储输入的序列,对其进行快速排序,数据结构C语言实现完整代码
时间: 2024-02-21 22:10:49 浏览: 36
好的,这里给出顺序表存储输入的序列,对其进行快速排序的完整C语言实现代码。其中,快速排序使用的是经典的 Hoare 算法。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int length;
} SqList;
int Partition(SqList *L, int low, int high) {
int pivot = L->data[low];
while (low < high) {
while (low < high && L->data[high] >= pivot) {
high--;
}
L->data[low] = L->data[high];
while (low < high && L->data[low] <= pivot) {
low++;
}
L->data[high] = L->data[low];
}
L->data[low] = pivot;
return low;
}
void QuickSort(SqList *L, int low, int high) {
if (low < high) {
int pivotpos = Partition(L, low, high);
QuickSort(L, low, pivotpos - 1);
QuickSort(L, pivotpos + 1, high);
}
}
int main() {
SqList L;
int n, i;
printf("Input the number of elements (<= %d): ", MAX_SIZE);
scanf("%d", &n);
if (n > MAX_SIZE) {
printf("Error: the number of elements is too large!\n");
return -1;
}
printf("Input %d elements: ", n);
for (i = 0; i < n; i++) {
scanf("%d", &L.data[i]);
}
L.length = n;
QuickSort(&L, 0, n - 1);
printf("The sorted sequence is: ");
for (i = 0; i < n; i++) {
printf("%d ", L.data[i]);
}
printf("\n");
return 0;
}
```
在该代码中,Partition 函数用于将顺序表 L 中下标在 low 到 high 之间的元素进行划分,返回枢轴元素最终所在位置。QuickSort 函数用于递归实现快速排序。最后,输入的序列被存储在顺序表 L 中,经过快速排序后,输出排序后的序列。
相关推荐
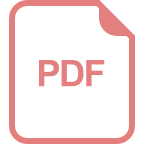
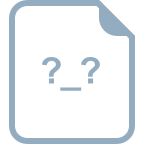
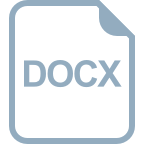














