C#winForm利用单例模式和多线程反复打印"你好"到界面上
时间: 2024-10-03 09:03:32 浏览: 83
在C# WinForms中,你可以结合单例模式(Singleton Pattern)来创建一个全局唯一的实例,然后使用多线程(Multithreading)来实现反复地、并发地将“你好”打印到界面控件上。以下是简化的步骤:
首先,我们需要创建一个单例类,它包含一个静态的Thread变量用于存储线程,并有一个锁定对象(如private static object _syncLock)来保证线程安全:
```csharp
public class GreetingDisplay : ISingleton
{
private static readonly GreetingDisplay instance;
private Thread printThread;
private GreetingDisplay() { }
// 单例获取方法
public static GreetingDisplay Instance
{
get
{
if (instance == null)
lock (_syncLock)
if (instance == null) instance = new GreetingDisplay();
return instance;
}
}
// 模拟打印方法,实际操作应放到后台线程
private void PrintHello()
{
for (;;)
{
Invoke(new Action(() => textBox.AppendText("你好\n"))); // 使用Invoke确保UI线程更新
System.Threading.Thread.Sleep(1000); // 每秒打印一次
}
}
// 启动打印线程
public void StartPrint()
{
if (printThread != null && !printThread.IsAlive)
printThread = new Thread(PrintHello);
printThread.Start();
}
// 停止打印
public void StopPrint()
{
printThread?.Abort(); // 如果线程还在运行,尝试停止
}
}
```
接下来,在你的WinForm中,你需要添加必要的事件处理和初始化:
```csharp
public partial class MainForm : Form
{
public MainForm()
{
InitializeComponent();
// 初始化单例并启动打印
GreetingDisplay.Instance.StartPrint();
}
protected override void OnClosing(CancelEventArgs e)
{
// 关闭应用前,先停止打印
GreetingDisplay.Instance.StopPrint();
base.OnClosing(e);
}
}
// 使用ISingleton接口来获取实例
interface ISingleton
{
GreetingDisplay Instance { get; }
}
```
当你打开这个WinForm应用程序时,“你好”就会每秒出现在文本框中,直到关闭程序。注意这里只是一个简化示例,实际应用中还需要处理异常和线程同步等问题。
阅读全文
相关推荐





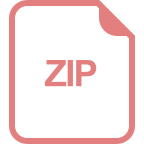












