C语言基于链式存储结构的图书信息表的排序代码
时间: 2024-09-25 08:13:40 浏览: 111
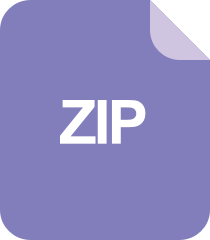
1.基于顺序存储结构的图书信息表的创建和输出 2..基于顺序存储结构的图书信息表的排序 3.基于顺序存储结构的图书信息表的修改
在C语言中,我们可以使用链表数据结构来存储图书信息,并实现排序功能。这里假设我们有一个包含图书ID、标题、作者等字段的简单链表节点结构体。为了对图书信息进行排序,常见的排序算法有插入排序、选择排序、归并排序等。下面是一个使用插入排序的例子:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct BookNode {
int id;
char title[50];
char author[50];
struct BookNode* next;
} BookNode;
// 插入排序函数
void insertionSort(BookNode** head) {
if (*head == NULL || (*head)->next == NULL) return; // 如果链表为空或只有一个元素,无需排序
BookNode* current = *head;
BookNode* temp;
while (current->next != NULL) { // 遍历链表
temp = current->next;
if (temp->id < current->id) { // 比较当前节点和下一个节点
// 交换节点位置
BookNode* temp_next = temp->next;
temp->next = current;
current->next = temp_next;
// 继续遍历
current = temp;
} else {
current = current->next;
}
}
}
// 添加新书到链表
void addBook(BookNode** head, int id, const char* title, const char* author) {
BookNode* newNode = (BookNode*)malloc(sizeof(BookNode));
newNode->id = id;
strcpy(newNode->title, title);
strcpy(newNode->author, author);
newNode->next = *head;
*head = newNode;
}
// 打印链表
void printList(BookNode* head) {
BookNode* temp = head;
while (temp != NULL) {
printf("ID: %d, Title: %s, Author: %s\n", temp->id, temp->title, temp->author);
temp = temp->next;
}
}
int main() {
// 初始化链表
BookNode* head = NULL;
// 添加一些书籍
addBook(&head, 5, "书名A", "作者A");
addBook(&head, 3, "书名B", "作者B");
addBook(&head, 1, "书名C", "作者C");
// 对图书信息按ID排序
insertionSort(&head);
// 打印排序后的链表
printList(head);
return 0;
}
```
阅读全文
相关推荐
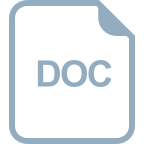


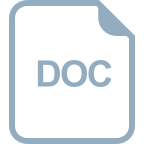
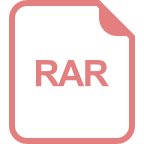
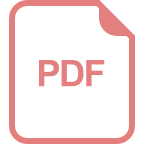
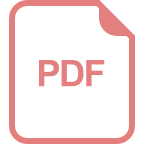
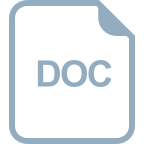
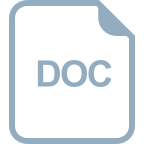
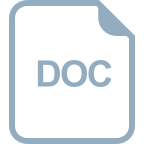
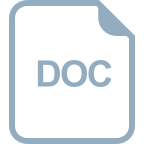
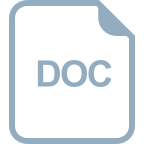
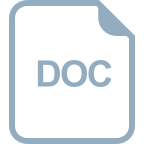
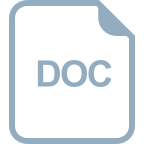