AttributeError: 'numpy.ndarray' object has no attribute 'str'
时间: 2023-10-02 11:12:39 浏览: 474
这个错误通常发生在尝试对一个 NumPy 数组对象调用字符串方法时。由于NumPy数组是多维的,不能直接使用`str()`方法进行转换。相反,您可以使用`numpy.ndarray.astype()`方法将数组转换为字符串类型。
下面是一个例子:
```python
import numpy as np
# 创建一个NumPy数组
arr = np.array([1, 2, 3])
# 将数组转换为字符串类型
arr_str = arr.astype(str)
# 打印转换后的字符串数组
print(arr_str)
```
请注意,这里的`arr_str`是一个新的字符串数组对象,而不是原始的NumPy数组。您可以根据需要使用`arr_str`进行进一步操作。
相关问题
AttributeError: 'numpy.ndarray' object has no attribute 'decode'
这个错误通常发生在尝试对numpy数组进行解码的时候。在Python 3中,字符串和字节类型是严格区分的,因此不能将字节数组直接解码为字符串。
如果你需要将numpy数组转换为字符串,可以使用numpy的tostring()方法将数组转换为字节类型,然后使用decode()方法将字节类型转换为字符串类型。
例如,假设你有一个numpy数组arr:
```
arr = numpy.array([1, 2, 3])
```
要将该数组转换为字符串,可以使用以下代码:
```
arr_bytes = arr.tostring()
arr_str = arr_bytes.decode('utf-8')
```
这将使用utf-8编码将字节数组转换为字符串。你可以根据需要使用不同的编码方式进行转换。
如何解决:AttributeError: 'numpy.ndarray' object has no attribute 'decode'
在 Python 中,`decode()` 方法是用于将 bytes 对象转换为字符串的方法。因此,如果你在一个 numpy 的 ndarray 上调用了 `decode()` 方法,就会出现 `AttributeError: 'numpy.ndarray' object has no attribute 'decode'` 的错误。
要解决这个错误,你需要确定哪个 ndarray 对象上调用了 `decode()` 方法。如果你可以确定 ndarray 对象中包含的是字符串类型的数据,那么你可以使用 numpy 的`astype()`方法将其转换为字符串类型:
```python
new_array = old_array.astype('str')
```
或者,你可以在调用 `decode()` 方法之前,先将 ndarray 对象转换为 bytes 类型。例如:
```python
bytes_array = np.ndarray.tobytes(ndarray_object)
decoded_string = bytes_array.decode('utf-8')
```
这样就可以避免 `AttributeError: 'numpy.ndarray' object has no attribute 'decode'` 的错误了。
阅读全文
相关推荐
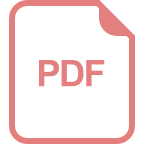
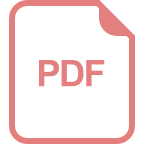














