如何将一张图像作为输入,并用这些代码计算出该图像的HOG特征,请给出完整示例
时间: 2024-04-15 21:30:17 浏览: 31
下面是一个完整示例,演示如何使用给定的代码计算一张图像的HOG特征:
```cpp
#include <iostream>
#include <opencv2/opencv.hpp>
struct DETECTION_ROW {
cv::Vec4f tlwh;
float feature[128];
};
float* get_hog_feature(cv::Mat img)
{
cv::HOGDescriptor hog = cv::HOGDescriptor(cvSize(20, 20), cvSize(10, 10), cvSize(5, 5), cvSize(5, 5), 9);
cv::resize(img, img, cv::Size(30, 30), (0, 0), (0, 0), cv::INTER_LINEAR);
std::vector<float> descriptors;
hog.compute(img, descriptors, cv::Size(20, 20), cv::Size(0, 0));
float* feature_float = (float*)malloc(descriptors.size() * sizeof(float));
assert(feature_float);
for (int i = 0; i < 128; i++)
{
feature_float[i] = descriptors[i * 2];
}
return feature_float;
}
bool getRectsHogFeature(const cv::Mat& img, std::vector<DETECTION_ROW>& d)
{
std::vector<cv::Mat> mats;
int feature_dim = 128;
for (DETECTION_ROW& dbox : d)
{
cv::Rect rc = cv::Rect(int(dbox.tlwh(0)), int(dbox.tlwh(1)), int(dbox.tlwh(2)), int(dbox.tlwh(3)));
rc.x = (rc.x >= 0 ? rc.x : 0);
rc.y = (rc.y >= 0 ? rc.y : 0);
rc.width = (rc.x + rc.width <= img.cols ? rc.width : (img.cols - rc.x));
rc.height = (rc.y + rc.height <= img.rows ? rc.height : (img.rows - rc.y));
cv::Mat mattmp = img(rc).clone();
float* feature_float = get_hog_feature(mattmp);
for (int i = 0; i < feature_dim; i++)
{
dbox.feature[i] = feature_float[i];
}
}
return true;
}
int main()
{
// 读取图像
cv::Mat img = cv::imread("image.jpg", cv::IMREAD_GRAYSCALE);
if (img.empty())
{
std::cout << "Failed to read image!" << std::endl;
return -1;
}
// 定义包含矩形框信息的容器
std::vector<DETECTION_ROW> d;
DETECTION_ROW dbox;
dbox.tlwh = cv::Vec4f(10, 10, 50, 50); // 示例矩形框信息
d.push_back(dbox);
// 计算图像的HOG特征
getRectsHogFeature(img, d);
// 打印第一个矩形框的HOG特征值
std::cout << "HOG features for the first detection:" << std::endl;
for (int i = 0; i < 128; i++)
{
std::cout << d[0].feature[i] << " ";
}
std::cout << std::endl;
return 0;
}
```
在这个示例中,首先读取一张图像,然后定义一个包含矩形框信息的容器 `d`,并向其中添加一个示例矩形框信息。
接下来,调用 `getRectsHogFeature` 函数,计算图像的HOG特征,并将特征存储到相应的数据结构中。
最后,打印第一个矩形框的HOG特征值。
请确保将示例图像文件 `image.jpg` 放在同一目录下,并使用适当的OpenCV版本进行编译和运行。
相关推荐
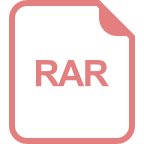
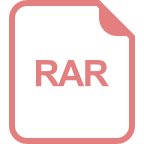
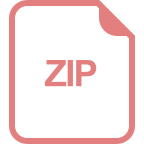









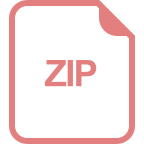
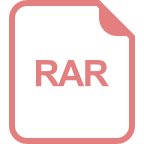
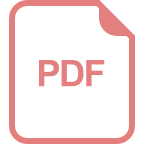
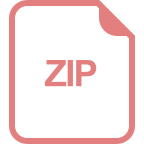
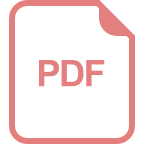
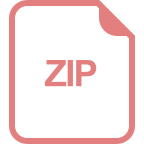