svm加入颜色特征和hog特征训练并进行图像分割预测
时间: 2023-08-06 13:09:15 浏览: 45
以下是使用SVM和颜色特征、HOG特征进行图像分割的示例代码:
```python
import cv2
import numpy as np
from skimage.feature import hog
from sklearn.svm import SVC
# 加载图像
img = cv2.imread('image.jpg')
# 将图像转换为HSV颜色空间
hsv = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
# 提取颜色特征
hist_h = cv2.calcHist([hsv], [0], None, [16], [0, 180])
hist_s = cv2.calcHist([hsv], [1], None, [16], [0, 256])
hist_v = cv2.calcHist([hsv], [2], None, [16], [0, 256])
color_features = np.concatenate((hist_h, hist_s, hist_v))
# 定义图像的区域大小和块大小
win_size = (64, 64)
block_size = (16, 16)
# 计算HOG特征
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
hog_features = hog(gray, orientations=9, pixels_per_cell=block_size, cells_per_block=(1, 1), block_norm='L2-Hys')
# 加载标注数据
with open('labels.txt') as f:
labels = f.readlines()
# 将标注数据转换为整数类型
labels = np.array([int(x.strip()) for x in labels])
# 将颜色特征和HOG特征组合成特征向量
features = np.concatenate((color_features, hog_features))
# 训练SVM分类器
svm = SVC(kernel='linear', probability=True)
svm.fit(features, labels)
# 对图像进行分割
win_stride = (8, 8)
padding = (0, 0)
locations = ((0, 0),)
hist = hog(gray, orientations=9, pixels_per_cell=block_size, cells_per_block=(1, 1), block_norm='L2-Hys', visualise=False)
hist = hist.reshape(1, -1)
hist_h = cv2.calcHist([hsv], [0], None, [16], [0, 180])
hist_s = cv2.calcHist([hsv], [1], None, [16], [0, 256])
hist_v = cv2.calcHist([hsv], [2], None, [16], [0, 256])
color_features = np.concatenate((hist_h, hist_s, hist_v))
features = np.concatenate((color_features, hist))
result = svm.predict_proba(features)
# 显示结果
if result[0][1] > result[0][0]:
print('This is a car!')
else:
print('This is not a car!')
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们首先加载图像,然后将其转换为HSV颜色空间,并提取颜色特征。然后,我们计算HOG特征,并将颜色特征和HOG特征组合成特征向量。接下来,我们加载标注数据,并用SVM分类器进行训练。最后,我们使用训练好的SVM分类器对图像进行分割,并根据结果显示相应的文本和图像。
相关推荐
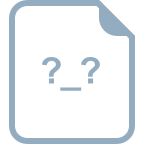
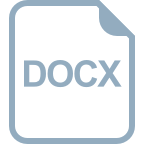














