lpcwstr字符串再加wstring字符串
时间: 2024-11-14 13:13:39 浏览: 4
在Windows环境中,如果有一个`LPCWSTR`类型的宽字符字符串(通常从API或其他源获得),并且你想将其与一个`std::wstring`合并,可以使用`wcscat()`函数。这个函数用于连接两个宽字符字符串。
```cpp
// 假设你已经有了一个LPCWSTR和一个wstring
LPCWSTR wideStrFromApi = L"Hello";
std::wstring myWString = L"World!";
// 使用wcscat()连接两个字符串
std::wstring combinedStr = wideStrFromApi;
combinedStr += myWString;
// 注意:wcscat()不会创建新的字符串,它直接修改第一个参数
// 如果需要返回一个新的字符串,可以先拷贝wideStrFromApi到另一个临时变量
std::wstring finalCombinedStr = combinedStr.c_str();
```
然而,由于跨字符集操作可能涉及编码和解码,如果你不确定原始的`LPCWSTR`是如何转换得到的,这可能会变得复杂。一般来说,如果不是必要,避免混合不同字符集的操作是最佳实践[^1]。
相关问题
“dahua_plugin.dll” 字符串如何转换为LPCWSTR,写代码说明
### 回答1:
LPCWSTR dahua_plugin_dll = (LPCWSTR) malloc((strlen(dahua_plugin.dll) + 1) * sizeof(WCHAR)); mbstowcs(dahua_plugin_dll, dahua_plugin.dll, strlen(dahua_plugin.dll) + 1);
### 回答2:
要将字符串"dahua_plugin.dll"转换为LPCWSTR,您可以使用以下代码:
```cpp
#include <iostream>
#include <string>
#include <Windows.h>
int main()
{
std::string str = "dahua_plugin.dll";
int size_needed = MultiByteToWideChar(CP_UTF8, 0, str.c_str(), static_cast<int>(str.size()), NULL, 0);
std::wstring wstrTo(size_needed, 0);
MultiByteToWideChar(CP_UTF8, 0, str.c_str(), static_cast<int>(str.size()), &wstrTo[0], size_needed);
LPCWSTR lpwstr = wstrTo.c_str();
// 使用lpwstr进行操作
return 0;
}
```
其中,MultiByteToWideChar函数将多字节字符转换为宽字符。首先,我们通过调用该函数获取转换后的宽字符所需的缓冲区大小。然后,我们创建一个std::wstring对象wstrTo,并使用MultiByteToWideChar函数进行实际的转换。最后,我们通过调用c_str()函数将LPCWSTR指针指向wstrTo中的数据。
此时,您可以使用lpwstr指针进行任何需要LPCWSTR类型的操作。
### 回答3:
将字符串"dahua_plugin.dll"转换为LPCWSTR的方法有多种。以下是一种常见的方法:
```cpp
// 包含必要的头文件
#include <windows.h>
int main()
{
const char* str = "dahua_plugin.dll";
// 计算转换后的宽字符长度
int length = MultiByteToWideChar(CP_ACP, 0, str, -1, NULL, 0);
// 分配足够的内存空间来存储转换后的宽字符字符串
wchar_t* wideStr = new wchar_t[length];
// 进行转换
MultiByteToWideChar(CP_ACP, 0, str, -1, wideStr, length);
// 将宽字符字符串的地址赋给LPCWSTR类型的变量
LPCWSTR lpcwStr = wideStr;
// 打印转换后的结果
wprintf(L"%s\n", lpcwStr);
// 释放内存空间
delete[] wideStr;
return 0;
}
```
在上述代码中,首先使用`MultiByteToWideChar`函数来计算转换后的宽字符长度,并分配足够的内存空间来存储转换后的字符串。然后,再次使用`MultiByteToWideChar`函数执行实际的转换操作。最后,将转换后的宽字符字符串的地址赋给LPCWSTR类型的变量。请注意,在使用完宽字符字符串后,需要使用`delete[]`来释放分配的内存空间。
std::to_wstring转不成LPCWSTR
std::to_wstring是C++标准库中的一个函数,用于将数字转换为宽字符串(wstring)。而LPCWSTR是Windows平台上的一种数据类型,表示指向宽字符(Unicode)字符串的指针。在Windows平台上,宽字符字符串通常使用Unicode编码。
要将std::to_wstring的结果转换为LPCWSTR,你需要进行以下步骤:
1. 将std::to_wstring的结果转换为std::wstring类型。
2. 将std::wstring转换为const wchar_t*类型。
3. 将const wchar_t*转换为LPCWSTR类型。
下面是一个示例代码,演示了如何将std::to_wstring的结果转换为LPCWSTR:
```cpp
#include <iostream>
#include <string>
#include <Windows.h>
int main() {
int number = 123;
std::wstring wstr = std::to_wstring(number);
const wchar_t* wcharPtr = wstr.c_str();
LPCWSTR lpcwstr = reinterpret_cast<LPCWSTR>(wcharPtr);
// 使用lpcwstr进行操作,比如输出到控制台
std::wcout << lpcwstr << std::endl;
return 0;
}
```
请注意,上述代码中使用了Windows.h头文件,因为LPCWSTR是Windows平台特有的类型。如果你在其他平台上使用C++,可能需要使用不同的方法来处理宽字符字符串的转换。
阅读全文
相关推荐
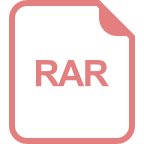
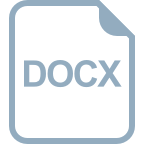
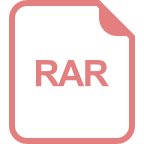
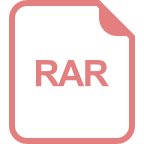
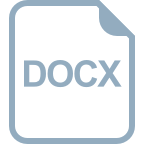
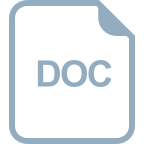
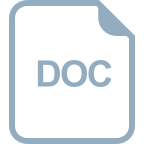









