用dev c++建立一个程序:从键盘上输入年月日,判断是这年的第几天
时间: 2024-12-09 20:18:42 浏览: 11
在Dev C++中创建一个简单的程序来计算给定日期是一年中的第几天,你可以按照以下步骤编写:
1. 首先,你需要包含必要的头文件,如`iostream`(用于输入/输出)和`ctime`(处理时间和日期)。
```cpp
#include <iostream>
#include <ctime>
```
2. 定义一个函数来获取给定日期是一年中的天数。这个函数可以接收年、月和日作为参数,并利用`mktime`和`difftime`函数来计算。
```cpp
int getDayOfYear(int year, int month, int day) {
struct tm date = {0};
date.tm_year = year - 1900; // 减去偏移量
date.tm_mon = month - 1;
date.tm_mday = day;
time_t t = mktime(&date);
time_t start_of_year = mktime(&({{year, 1, 1, 0, 0, 0, 0, 0, 0}}));
return static_cast<int>(difftime(t, start_of_year) + 1);
}
```
3. 在主函数`main()`中,提示用户输入年、月、日,然后调用上述函数并输出结果。
```cpp
int main() {
int year, month, day;
std::cout << "请输入年份: ";
std::cin >> year;
std::cout << "请输入月份(1-12): ";
std::cin >> month;
std::cout << "请输入日期(1-31): ";
std::cin >> day;
if (month > 12 || day > getDaysInMonth(year, month)) { // 检查输入是否有效
std::cerr << "无效的日期。\n";
return 1;
}
int days_in_year = getDayOfYear(year, month, day);
std::cout << "该日期是一年中的第" << days_in_year << "天。\n";
return 0;
}
// 辅助函数,获取给定月份有多少天
int getDaysInMonth(int year, int month) {
switch (month) {
case 2: {
if (is_leap_year(year)) return 29;
else return 28;
}
case 4: case 6: case 9: case 11: return 30;
default: return 31;
}
}
bool is_leap_year(int year) {
return year % 4 == 0 && (year % 100 != 0 || year % 400 == 0);
}
```
4. 编译并运行你的程序,注意输入的日期有效性检查(比如闰年二月有29天)。
阅读全文
相关推荐
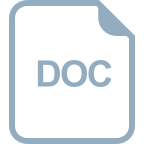
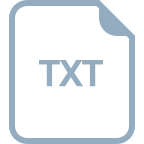
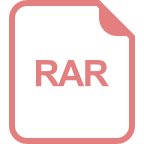
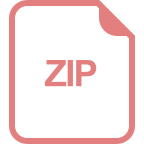
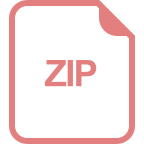
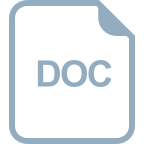
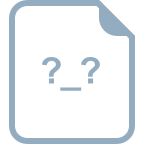
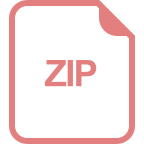
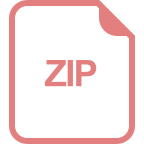
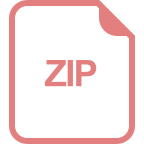
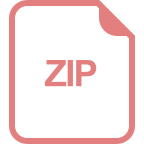
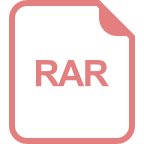
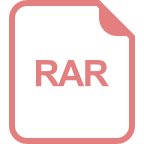
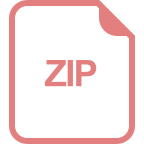