编写一个程序,先输入字符个数,再输入各个字符的权值,请以这些字符为叶子建立赫夫曼树,再求出其赫夫曼编码并打印
时间: 2024-11-09 12:18:06 浏览: 15
赫夫曼树(Huffman Tree),也叫最优二叉树,是一种自底向上的贪心算法用于构建最小带权路径长度的二叉树,常用于数据压缩。为了编写这样一个程序,你需要遵循以下步骤:
1. **获取用户输入**:
- 让用户输入字符的数量和每个字符对应的权值。你可以用字典或列表来存储这些信息。
```python
characters = {}
num_chars = int(input("请输入字符总数:"))
for i in range(num_chars):
char = input(f"请输入第{i+1}个字符:")
weight = float(input(f"请输入'{char}'的权重:"))
characters[char] = weight
```
2. **构造哈夫曼树**:
使用优先队列(通常可以用堆数据结构实现)来存储字符及其权重,并按照贪心策略合并权值较小的两个节点,直到只剩下一个节点为止。
```python
import heapq
def build_huffman_tree(characters):
heap = [[weight, [char, ""]] for char, weight in characters.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
combined_weight = left[0] + right[0]
combined_char = "(" + left[1][1:] + "|" + right[1][1:] + ")"
heapq.heappush(heap, [combined_weight, combined_char])
return heap[0][1]
huff_tree = build_huffman_tree(characters)
```
3. **生成赫夫曼编码**:
遍历赫夫曼树,从根开始,如果遇到的是字符节点,则添加当前节点作为前缀到编码;如果是左孩子节点,则编码加"0",如果是右孩子节点,则编码加"1"。
```python
def generate_huffman_codes(node, code=""):
if isinstance(node, str): # 到达叶节点,返回编码
return {node: code}
else:
left_code = generate_huffman_codes(node[0], code + "0")
right_code = generate_huffman_codes(node[1], code + "1")
return {k: v for k, v in left_code.items() | right_code.items()} # 合并两个子树的编码
huffman_codes = generate_huffman_codes(huff_tree)
```
4. **打印赫夫曼编码**:
最后,遍历编码字典并打印结果。
```python
for char, code in huffman_codes.items():
print(f"'{char}': {code}")
```
阅读全文
相关推荐
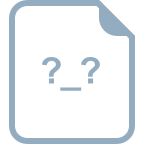
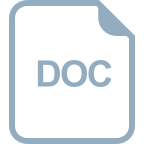
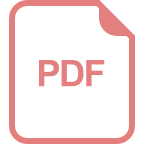
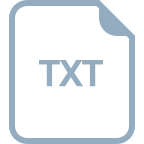
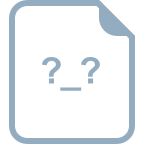
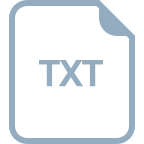
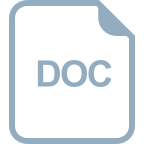










