用C语言写一段代码:明明生成了 n个1到500之间的随机整数,删除重复的数字,再把剩下的数字从小到大排序,输出排好的顺序
时间: 2024-10-10 08:08:40 浏览: 31
在C语言中,我们可以使用`<stdlib.h>`库的`rand()`函数生成随机数,`<time.h>`库的`srand()`和`clock()`函数初始化随机数种子,并使用`<stdio.h>`库处理输入输出。这里是一个简单的示例,它首先生成随机数并存储在一个数组中,然后使用`qsort()`函数对数组进行排序,最后输出排序后的结果:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 函数声明用于排序
void swap(int* a, int* b) {
int t = *a;
*a = *b;
*b = t;
}
int compare(const void* a, const void* b) {
return (*(const int*)a - *(const int*)b);
}
// 主函数
int main() {
srand(time(0)); // 初始化随机数种子
int n;
printf("Enter the number of random integers (1 to 500): ");
scanf("%d", &n);
if (n > 500 || n <= 0) {
printf("Error: Number out of range. Please enter between 1 and 500.\n");
return 1;
}
// 创建一个大小为n的动态数组
int* numbers = malloc(n * sizeof(int));
if (!numbers) {
perror("Memory allocation failed.");
return 1;
}
for (int i = 0; i < n; ++i) {
numbers[i] = rand() % 500 + 1; // 生成1到500之间的随机数
}
// 删除重复元素
int count = 0;
for (int i = 0; i < n; ++i) {
int is_unique = 1;
for (int j = 0; j < i && is_unique; ++j) {
if (numbers[i] == numbers[j]) {
is_unique = 0;
}
}
if (is_unique) {
numbers[count++] = numbers[i];
}
}
n = count;
// 对剩余的元素进行排序
qsort(numbers, n, sizeof(int), compare);
// 输出排序后的结果
printf("Sorted unique numbers from 1 to 500:\n");
for (int i = 0; i < n; ++i) {
printf("%d ", numbers[i]);
}
printf("\n");
free(numbers); // 释放内存
return 0;
}
```
阅读全文
相关推荐
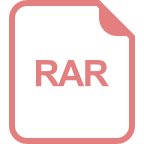
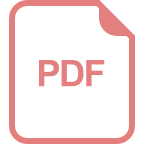
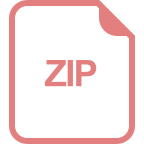















