编写一个算法,检测一个C++源程序文件中的括号是否配对,包括“()[]{}”这三类括号。需要注意的是,单个括号字符,字符串中的括号,注释中的括号均不列入检测范围。用C编写程序实现该算法。选做内容:标注所有不配对括号的位置。
时间: 2024-10-06 19:05:11 浏览: 48
在C语言中,你可以使用栈数据结构来检测C++源程序文件中的括号是否配对。下面是一个简单的算法步骤:
1. 初始化一个空栈(Stack)用于存储左括号。
2. 遍历源程序文件中的每个字符:
a. 如果遇到左括号(如'('、'['或'{'),将其压入栈中。
b. 如果遇到右括号(如')'、']'或'}'):
i. 检查栈顶元素是否对应当前右括号。例如,如果遇到了')',检查栈顶是不是 '(';如果是 ']',检查栈顶是不是 '[' 等。
ii. 如果匹配,弹出栈顶元素;如果不匹配,说明有不配对的括号。
c. 如果遍历到字符串结束,但栈未为空,表示存在不配对的括号。
3. 标注不配对括号位置:在遍历过程中,当发现不匹配的情况,记录下当前位置(可以用行号和列号等信息表示)。
这是一个基础的实现思路,如果你需要在程序中直接运行这个算法并输出结果,你需要将这个逻辑封装成一个函数,并处理文件输入、输出以及错误处理。以下是伪代码形式:
```c
#include <stdio.h>
#include <stdbool.h>
// Stack data structure with push and pop operations
typedef struct {
char *items;
int top;
int size;
} Stack;
void push(Stack *s, char ch) {
// Implement stack push
}
char pop(Stack *s) {
// Implement stack pop
}
bool is_balanced(char *str) {
Stack s;
for (int i = 0; str[i] != '\0'; ++i) {
if (str[i] == '(' || str[i] == '[' || str[i] == '{')
push(&s, str[i]);
else if (str[i] == ')' && s.top > 0 && s.items[s.top - 1] == '(')
pop(&s);
else if (str[i] == ']' && s.top > 0 && s.items[s.top - 1] == '[')
pop(&s);
else if (str[i] == '}' && s.top > 0 && s.items[s.top - 1] == '{')
pop(&s);
else if (s.top > 0) // Unmatched closing bracket found
return false;
}
return s.top == 0; // No unmatched opening brackets at end
}
// Function to find unbalanced pairs and their positions
void find_unbalanced_positions(char *str) {
// Implement this function to store the positions in an appropriate format
}
int main() {
char *file_content; // Read file content here
if (!is_balanced(file_content)) {
find_unbalanced_positions(file_content); // Call the above function
// Output the unbalanced positions
}
return 0;
}
```
阅读全文
相关推荐
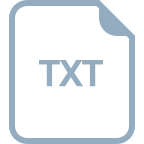
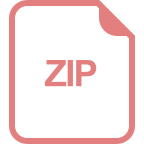
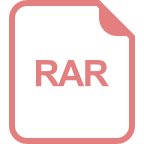

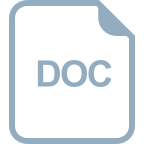
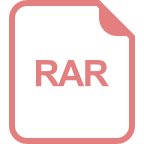
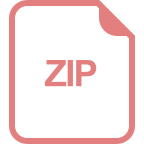
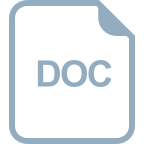

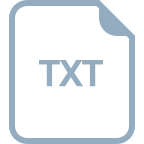
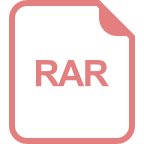
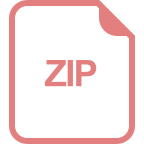
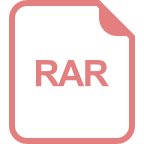
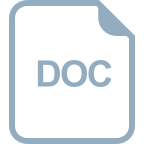
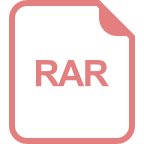
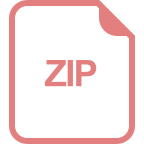
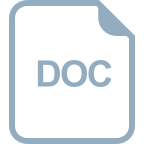
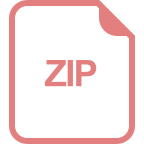