matlab四叉树碰撞
时间: 2024-02-17 13:58:16 浏览: 23
四叉树碰撞检测是一种常用的碰撞检测算法,它通过将空间划分为四个象限来提高碰撞检测的效率。在MATLAB中,可以使用四叉树来实现碰撞检测。以下是一个MATLAB的四叉树碰撞检测的示例代码:
```matlab
classdef QuadtreeNode
properties
x
y
width
height
objects
children
end
methods
function obj = QuadtreeNode(x, y, width, height)
obj.x = x;
obj.y = y;
obj.width = width;
obj.height = height;
obj.objects = [];
obj.children = [];
end
function insert(obj, object)
if ~obj.isLeaf()
for i = 1:4
if obj.children(i).contains(object)
obj.children(i).insert(object);
return;
end
end
end
obj.objects = [obj.objects, object];
if obj.isOverCapacity() && obj.isLeaf()
obj.split();
end
end
function split(obj)
halfWidth = obj.width / 2;
halfHeight = obj.height / 2;
obj.children = [
QuadtreeNode(obj.x, obj.y, halfWidth, halfHeight),
QuadtreeNode(obj.x + halfWidth, obj.y, halfWidth, halfHeight),
QuadtreeNode(obj.x, obj.y + halfHeight, halfWidth, halfHeight),
QuadtreeNode(obj.x + halfWidth, obj.y + halfHeight, halfWidth, halfHeight)
];
for i = 1:length(obj.objects)
for j = 1:4
if obj.children(j).contains(obj.objects(i))
obj.children(j).insert(obj.objects(i));
break;
end
end
end
obj.objects = [];
end
function result = isLeaf(obj)
result = isempty(obj.children);
end
function result = isOverCapacity(obj)
result = length(obj.objects) > 4;
end
function result = contains(obj, object)
result = object.x >= obj.x && object.x <= obj.x + obj.width && object.y >= obj.y && object.y <= obj.y + obj.height;
end
function result = query(obj, range)
result = [];
if ~obj.intersects(range)
return;
end
for i = 1:length(obj.objects)
if obj.objects(i).intersects(range)
result = [result, obj.objects(i)];
end
end
if ~obj.isLeaf()
for i = 1:4
result = [result, obj.children(i).query(range)];
end
end
end
function result = intersects(obj, range)
result = obj.x < range.x + range.width && obj.x + obj.width > range.x && obj.y < range.y + range.height && obj.y + obj.height > range.y;
end
end
end
classdef QuadtreeObject
properties
x
y
width
height
end
methods
function obj = QuadtreeObject(x, y, width, height)
obj.x = x;
obj.y = y;
obj.width = width;
obj.height = height;
end
function result = intersects(obj, range)
result = obj.x < range.x + range.width && obj.x + obj.width > range.x && obj.y < range.y + range.height && obj.y + obj.height > range.y;
end
end
end
% 创建一个四叉树节点
root = QuadtreeNode(0, 0, 100, 100);
% 创建一些对象并插入到四叉树中
object1 = QuadtreeObject(10, 10, 20, 20);
object2 = QuadtreeObject(30, 30, 20, 20);
object3 = QuadtreeObject(60, 60, 20, 20);
root.insert(object1);
root.insert(object2);
root.insert(object3);
% 查询与指定范围相交的对象
range = QuadtreeObject(0, 0, 50, 50);
result = root.query(range);
% 输出查询结果
for i = 1:length(result)
disp(['Object ', num2str(i), ': (', num2str(result(i).x), ', ', num2str(result(i).y), ')']);
end
```
这段代码创建了一个QuadtreeNode类和一个QuadtreeObject类,用于表示四叉树节点和对象。通过调用QuadtreeNode的insert方法,可以将对象插入到四叉树中。然后,可以使用QuadtreeNode的query方法查询与指定范围相交的对象。
相关推荐
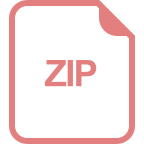














