你好,getline和string
时间: 2024-12-13 19:10:41 浏览: 4
你好!`getline`和`string`是C++中常用的字符串处理工具。
1. **`getline`**:
`getline`是一个函数,用于从输入流中读取一行文本。它通常用于从标准输入(如键盘)或文件输入中读取整行数据。`getline`函数有两种常见的形式:
- 使用`std::cin`:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str;
std::cout << "Enter a string: ";
std::getline(std::cin, str);
std::cout << "You entered: " << str << std::endl;
return 0;
}
```
- 使用文件流:
```cpp
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cout << "Unable to open file";
}
return 0;
}
```
2. **`string`**:
`string`是C++标准库中的一个类,用于处理字符串。它提供了许多有用的成员函数和方法,使得字符串操作更加方便和高效。例如:
- 字符串连接:
```cpp
std::string str1 = "Hello, ";
std::string str2 = "World!";
std::string result = str1 + str2; // "Hello, World!"
```
- 字符串长度:
```cpp
std::string str = "Hello";
size_t length = str.length(); // 5
```
- 子字符串:
```cpp
std::string str = "Hello, World!";
std::string sub = str.substr(7, 5); // "World"
```
- 查找子字符串:
```cpp
std::string str = "Hello, World!";
size_t pos = str.find("World"); // 7
```
`getline`和`string`结合使用可以方便地从输入中读取和处理字符串数据。
阅读全文
相关推荐
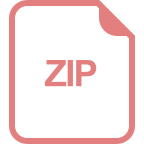
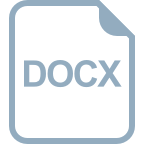
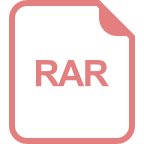
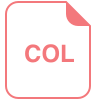
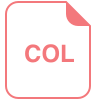











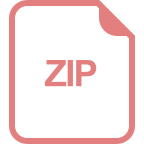
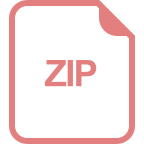
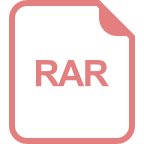