unordered_map 键值为char数组
时间: 2024-02-15 15:00:47 浏览: 180
unordered_map 是C++标准库中的一个关联容器,它提供了一种键值对的映射关系。在unordered_map中,键和值可以是任意类型,包括char数组。
使用unordered_map来存储char数组作为键时,需要自定义一个哈希函数和一个相等比较函数,以确保正确的键值对映射。下面是一个示例:
```cpp
#include <iostream>
#include <unordered_map>
#include <cstring>
// 自定义哈希函数
struct CharArrayHash {
std::size_t operator()(const char* arr) const {
std::size_t hash = 0;
for (std::size_t i = 0; i < std::strlen(arr); ++i) {
hash = hash * 31 + arr[i];
}
return hash;
}
};
// 自定义相等比较函数
struct CharArrayEqual {
bool operator()(const char* arr1, const char* arr2) const {
return std::strcmp(arr1, arr2) == 0;
}
};
int main() {
std::unordered_map<const char*, int, CharArrayHash, CharArrayEqual> myMap;
// 插入键值对
myMap["key1"] = 1;
myMap["key2"] = 2;
myMap["key3"] = 3;
// 访问值
std::cout << "Value of key2: " << myMap["key2"] << std::endl;
return 0;
}
```
在上面的示例中,我们自定义了一个哈希函数 `CharArrayHash` 和一个相等比较函数 `CharArrayEqual`,并将它们作为模板参数传递给 `unordered_map`。然后,我们可以使用char数组作为键来插入和访问值。
阅读全文
相关推荐
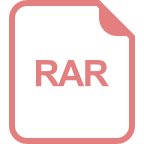
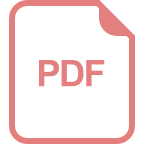
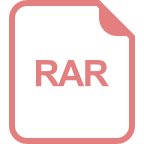
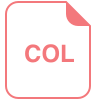
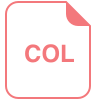
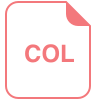
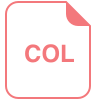
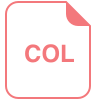
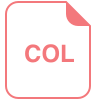
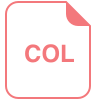
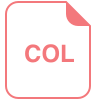
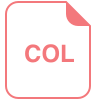
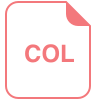
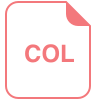
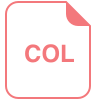
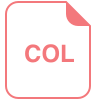
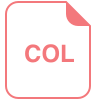
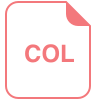
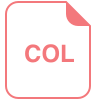