qt ffmpeg推拉流 程序
时间: 2023-11-08 07:02:22 浏览: 123
对于Qt和FFmpeg的推拉流程序,你可以使用Qt的多媒体模块和FFmpeg库来实现。下面是一个简单的示例程序,演示了如何使用Qt和FFmpeg实现推拉流:
```cpp
#include <QCoreApplication>
#include <QMediaPlayer>
extern "C" {
#include <libavformat/avformat.h>
}
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 初始化FFmpeg
av_register_all();
// 创建AVFormatContext
AVFormatContext *formatContext = avformat_alloc_context();
// 打开输入流
if (avformat_open_input(&formatContext, "input.mp4", nullptr, nullptr) != 0) {
avformat_free_context(formatContext);
qDebug() << "无法打开输入流";
return -1;
}
// 读取流信息
if (avformat_find_stream_info(formatContext, nullptr) < 0) {
avformat_close_input(&formatContext);
qDebug() << "无法读取流信息";
return -1;
}
// 打开输出流
AVFormatContext *outputFormatContext = nullptr;
if (avformat_alloc_output_context2(&outputFormatContext, nullptr, "flv", "output.flv") < 0) {
avformat_close_input(&formatContext);
qDebug() << "无法打开输出流";
return -1;
}
// 遍历输入流
for (unsigned int i = 0; i < formatContext->nb_streams; ++i) {
AVStream *inputStream = formatContext->streams[i];
AVStream *outputStream = avformat_new_stream(outputFormatContext, inputStream->codec->codec);
if (avcodec_copy_context(outputStream->codec, inputStream->codec) < 0) {
avformat_close_input(&formatContext);
avformat_free_context(outputFormatContext);
qDebug() << "无法复制编解码器上下文";
return -1;
}
outputStream->codec->codec_tag = 0;
if (outputFormatContext->oformat->flags & AVFMT_GLOBALHEADER) {
outputStream->codec->flags |= AV_CODEC_FLAG_GLOBAL_HEADER;
}
}
// 打开输出流
if (!(outputFormatContext->oformat->flags & AVFMT_NOFILE)) {
if (avio_open(&outputFormatContext->pb, "output.flv", AVIO_FLAG_WRITE) < 0) {
avformat_close_input(&formatContext);
avformat_free_context(outputFormatContext);
qDebug() << "无法打开输出文件";
return -1;
}
}
// 写入流头部信息
if (avformat_write_header(outputFormatContext, nullptr) < 0) {
avformat_close_input(&formatContext);
avformat_free_context(outputFormatContext);
qDebug() << "无法写入输出流头部信息";
return -1;
}
// 推流数据
AVPacket packet;
while (av_read_frame(formatContext, &packet) >= 0) {
AVStream *inputStream = formatContext->streams[packet.stream_index];
AVStream *outputStream = outputFormatContext->streams[packet.stream_index];
// 设置时间基
av_packet_rescale_ts(&packet, inputStream->time_base, outputStream->time_base);
packet.pts = packet.dts;
// 写入数据包
if (av_interleaved_write_frame(outputFormatContext, &packet) < 0) {
av_packet_unref(&packet);
avformat_close_input(&formatContext);
avformat_free_context(outputFormatContext);
qDebug() << "无法写入数据包";
return -1;
}
av_packet_unref(&packet);
}
// 写入流尾部信息
av_write_trailer(outputFormatContext);
// 关闭输入流和输出流
avformat_close_input(&formatContext);
avformat_free_context(outputFormatContext);
return a.exec();
}
```
请确保已正确安装FFmpeg,并将`input.mp4`替换为实际的输入文件名,将`output.flv`替换为实际的输出文件名。
阅读全文
相关推荐
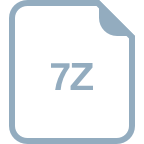
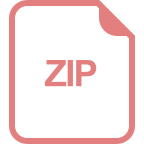
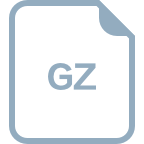
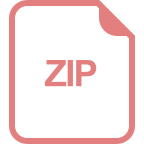
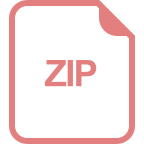
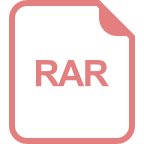
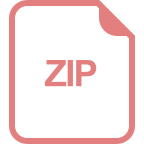
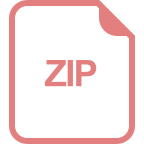
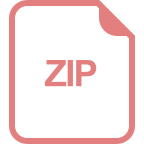
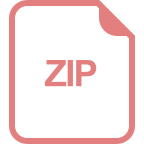
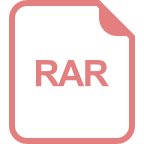
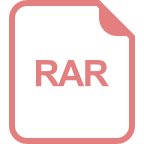
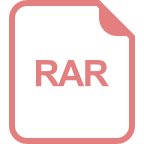
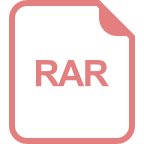