完成以下操作并给出完整代码:1、编写程序通过注解及扫描方式实现容器的对象初始化 2、 使用spring与springmvc集成的方式改造用户管理系统
时间: 2024-12-21 22:21:22 浏览: 4
要通过注解和扫描方式实现Spring容器的对象初始化,你可以利用Spring的`@Component`或`@Service`等注解标记需要管理的类,然后使用`@Autowired`注解自动注入依赖。这里我为你提供一个简单的例子:
```java
import org.springframework.stereotype.Component;
// 使用@Component注解声明这是一个Spring Bean
@Component
public class ExampleBean {
// 假设我们有一个属性需要注入
private AnotherBean anotherBean;
// 使用@Autowired注解自动注入另一个bean
@Autowired
public void setAnotherBean(AnotherBean anotherBean) {
this.anotherBean = anotherBean;
}
// 这里可以有你的业务逻辑
public void doSomething() {
anotherBean.doSomethingElse();
}
}
// 类似地,对于其他需要管理的bean,也添加@Component注解
@Component
public class AnotherBean {
// ... (同样可以添加@Autowired注解进行依赖注入)
}
```
要使用Spring与Spring MVC集成,首先确保已经添加了Spring Web的相关依赖。接下来,你需要配置Spring MVC的Controller、视图解析器和前端控制器(如DispatcherServlet)。这里是一个基本的例子:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class UserController {
private final UserService userService;
// 使用@Autowired自动注入UserService
@Autowired
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/users")
public List<User> getUsers() {
return userService.getAllUsers(); // 调用UserService的方法获取数据
}
}
// 配置UserService,可能是一个接口或实现了某个接口的类
@Service
public interface UserService {
List<User> getAllUsers();
}
// 用户Service的具体实现
@Service
public class UserServiceImpl implements UserService {
// ...
}
```
记得要在web.xml或application.properties(如果你使用的是Spring Boot)文件中配置Spring MVC的启动。
阅读全文
相关推荐
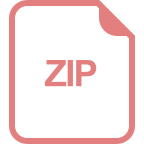
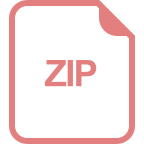
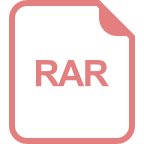
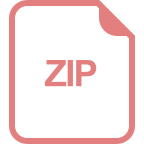
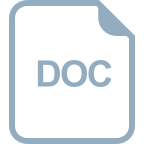
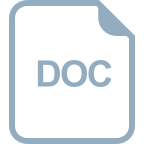
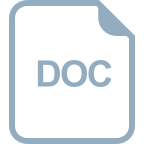
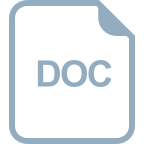
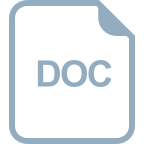
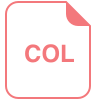
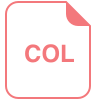
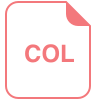
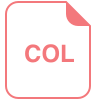
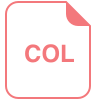
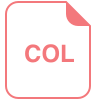
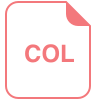
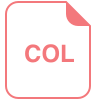
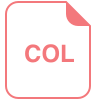
